for thread in tqdm(thread_list): thread.start() while threading.active_count() > thread_max: time.sleep(0.001) 请帮我用线程池改进这段代码
时间: 2023-08-22 20:05:19 浏览: 177
好的,请先确保安装了 Python 的 `concurrent.futures` 模块。
使用线程池改进这段代码,可以通过如下步骤实现:
1. 导入 `concurrent.futures` 模块。
2. 创建一个线程池对象,可以使用 `ThreadPoolExecutor` 类来创建线程池对象。
3. 将要执行的函数和参数打包成任务,提交给线程池对象的 `submit()` 方法。
4. 使用 `as_completed()` 函数来获取已完成的任务,并执行相应的操作。
下面是改进后的代码:
```python
import concurrent.futures
from tqdm import tqdm
def worker(thread):
thread.start()
return thread
thread_list = [...] # 线程列表
thread_max = ... # 最大线程数
with concurrent.futures.ThreadPoolExecutor(max_workers=thread_max) as executor:
future_to_thread = {executor.submit(worker, thread): thread for thread in thread_list}
for future in concurrent.futures.as_completed(future_to_thread):
# 对已完成的任务进行相应操作
pass
```
其中,`ThreadPoolExecutor(max_workers=thread_max)` 创建线程池对象,`executor.submit(worker, thread)` 提交任务,`as_completed(future_to_thread)` 获取已完成的任务。
需要注意的是,使用线程池时,任务的执行顺序可能会发生变化,因此需要根据具体需求进行相应的处理。
阅读全文
相关推荐
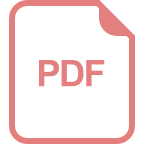

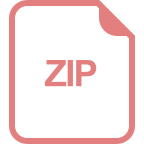
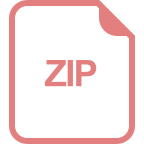
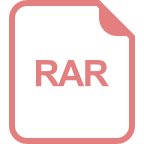
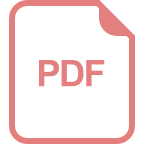
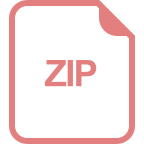
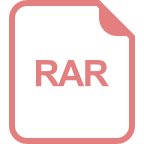
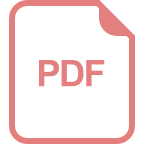
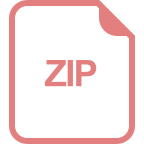
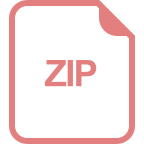
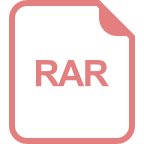
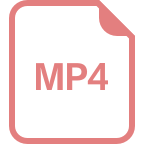
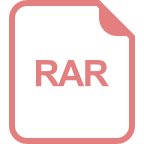
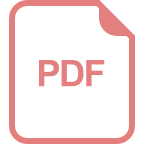