recyclerview分页smart kotlin
时间: 2023-07-21 19:02:45 浏览: 53
对于在 RecyclerView 中实现分页加载的功能,你可以使用 SmartRefreshLayout 库来实现。下面是使用 Kotlin 语言实现 RecyclerView 分页加载的基本步骤:
1. 首先,在你的项目中添加 SmartRefreshLayout 的依赖。在你的 build.gradle 文件中添加以下代码:
```kotlin
implementation 'com.scwang.smart:refresh-layout-kernel:2.0.3'
implementation 'com.scwang.smart:refresh-layout-kernel:2.0.3'
```
2. 在你的 XML 布局文件中添加 SmartRefreshLayout 和 RecyclerView:
```xml
<com.scwang.smartrefresh.layout.SmartRefreshLayout
android:id="@+id/refreshLayout"
android:layout_width="match_parent"
android:layout_height="match_parent">
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/recyclerView"
android:layout_width="match_parent"
android:layout_height="match_parent" />
</com.scwang.smartrefresh.layout.SmartRefreshLayout>
```
3. 在你的 Activity 或 Fragment 中设置 RecyclerView 的适配器,并初始化 SmartRefreshLayout:
```kotlin
val recyclerView: RecyclerView = findViewById(R.id.recyclerView)
val refreshLayout: SmartRefreshLayout = findViewById(R.id.refreshLayout)
val adapter = YourAdapter()
recyclerView.adapter = adapter
refreshLayout.setOnRefreshListener {
// 下拉刷新逻辑
// 清空数据,重新加载第一页数据
}
refreshLayout.setOnLoadMoreListener {
// 上拉加载逻辑
// 加载下一页数据,更新适配器
}
// 初始化时自动刷新
refreshLayout.autoRefresh()
```
4. 在上拉加载和下拉刷新的回调中,你可以进行数据的加载和更新逻辑。例如,在上拉加载的回调中,你可以请求下一页的数据,并将数据添加到适配器中:
```kotlin
fun loadMoreData(page: Int) {
// 请求下一页数据
// 将数据添加到适配器中
adapter.addData(newData)
// 加载完成后,调用 finishLoadMore() 方法
refreshLayout.finishLoadMore()
}
```
这就是使用 SmartRefreshLayout 实现 RecyclerView 分页加载的简单示例。你可以根据自己的需求进行定制和扩展。希望能对你有所帮助!
相关推荐
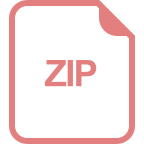














