使用Android实现,商品推荐页面有四个商品跳转到其中一个商品的详情页面,跳转使用intent,详情页面包括商品的名称、价格、介绍。推荐页面四个商品使用的gridview与fragment。详情页的activity与fragment也要给出
时间: 2024-02-06 21:10:54 浏览: 25
首先,我们需要在推荐页面的布局文件中添加一个GridView来显示四个商品。我们可以使用以下代码:
```xml
<GridView
android:id="@+id/grid_view"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:numColumns="2"
android:horizontalSpacing="10dp"
android:verticalSpacing="10dp"
android:padding="10dp" />
```
接下来,我们需要创建一个Fragment来显示GridView。我们可以使用以下代码:
```java
public class ProductFragment extends Fragment {
private GridView mGridView;
public ProductFragment() {
// Required empty public constructor
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.fragment_product, container, false);
mGridView = view.findViewById(R.id.grid_view);
// Set adapter for GridView
ProductAdapter adapter = new ProductAdapter(getActivity(), getProductList());
mGridView.setAdapter(adapter);
// Set click listener for GridView items
mGridView.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
// Launch ProductDetailActivity with selected product
Intent intent = new Intent(getActivity(), ProductDetailActivity.class);
intent.putExtra("product", getProductList().get(position));
startActivity(intent);
}
});
return view;
}
// Method to get list of products
private List<Product> getProductList() {
List<Product> productList = new ArrayList<>();
productList.add(new Product("Product 1", "$10.00", "Product 1 description."));
productList.add(new Product("Product 2", "$20.00", "Product 2 description."));
productList.add(new Product("Product 3", "$30.00", "Product 3 description."));
productList.add(new Product("Product 4", "$40.00", "Product 4 description."));
return productList;
}
}
```
在这个Fragment中,我们设置了一个ProductAdapter来显示GridView中的商品,并在点击GridView项时启动ProductDetailActivity。
接下来,我们需要创建一个ProductAdapter来显示GridView中的商品。我们可以使用以下代码:
```java
public class ProductAdapter extends ArrayAdapter<Product> {
public ProductAdapter(Context context, List<Product> products) {
super(context, 0, products);
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
if (convertView == null) {
convertView = LayoutInflater.from(getContext()).inflate(R.layout.item_product, parent, false);
}
Product product = getItem(position);
TextView nameTextView = convertView.findViewById(R.id.name_text_view);
TextView priceTextView = convertView.findViewById(R.id.price_text_view);
nameTextView.setText(product.getName());
priceTextView.setText(product.getPrice());
return convertView;
}
}
```
在这个ProductAdapter中,我们设置了一个item_product布局文件来显示GridView中的每个商品的名称和价格。
现在,我们需要创建一个ProductDetailActivity来显示选定商品的详细信息。我们可以使用以下代码:
```java
public class ProductDetailActivity extends AppCompatActivity {
private Product mProduct;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_product_detail);
// Get selected product from intent
mProduct = getIntent().getParcelableExtra("product");
// Set title of activity to product name
setTitle(mProduct.getName());
// Set product details
TextView nameTextView = findViewById(R.id.name_text_view);
TextView priceTextView = findViewById(R.id.price_text_view);
TextView descriptionTextView = findViewById(R.id.description_text_view);
nameTextView.setText(mProduct.getName());
priceTextView.setText(mProduct.getPrice());
descriptionTextView.setText(mProduct.getDescription());
}
}
```
在这个ProductDetailActivity中,我们获取从推荐页面传递的选定商品,并将其详细信息显示在屏幕上。
最后,我们需要创建一个fragment_product布局文件来显示ProductFragment中的GridView,以及一个item_product布局文件来显示每个商品的名称和价格,以及一个activity_product_detail布局文件来显示选定商品的详细信息。我们可以使用以下代码:
fragment_product.xml:
```xml
<GridView
android:id="@+id/grid_view"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:numColumns="2"
android:horizontalSpacing="10dp"
android:verticalSpacing="10dp"
android:padding="10dp" />
```
item_product.xml:
```xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="10dp">
<TextView
android:id="@+id/name_text_view"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:textSize="18sp"
android:textStyle="bold" />
<TextView
android:id="@+id/price_text_view"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="18sp"
android:textStyle="bold" />
</LinearLayout>
```
activity_product_detail.xml:
```xml
<ScrollView xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:padding="10dp">
<TextView
android:id="@+id/name_text_view"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="24sp"
android:textStyle="bold" />
<TextView
android:id="@+id/price_text_view"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="18sp"
android:textStyle="bold" />
<TextView
android:id="@+id/description_text_view"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="18sp" />
</LinearLayout>
</ScrollView>
```
现在,我们已经完成了Android应用程序中商品推荐页面和商品详情页面的创建。
相关推荐
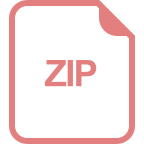
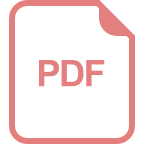
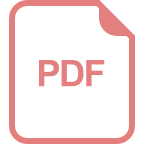















