用java 编写一个凸包算法的工具类
时间: 2023-09-02 16:12:26 浏览: 50
以下是使用 Java 编写的凸包算法工具类:
```java
import java.util.ArrayList;
import java.util.Comparator;
import java.util.List;
import java.util.Stack;
public class ConvexHull {
public static List<Point> getConvexHull(List<Point> points) {
if (points.size() < 3) {
return null;
}
// 找到y值最小的点,如果有多个,取x值最小的那个
Point minYPoint = points.get(0);
for (int i = 1; i < points.size(); i++) {
Point point = points.get(i);
if (point.y < minYPoint.y || (point.y == minYPoint.y && point.x < minYPoint.x)) {
minYPoint = point;
}
}
// 将所有点按极角排序
List<Point> sortedPoints = new ArrayList<>(points);
sortedPoints.remove(minYPoint);
sortedPoints.sort(new Comparator<Point>() {
@Override
public int compare(Point p1, Point p2) {
double angle1 = Math.atan2(p1.y - minYPoint.y, p1.x - minYPoint.x);
double angle2 = Math.atan2(p2.y - minYPoint.y, p2.x - minYPoint.x);
if (angle1 < angle2) {
return -1;
} else if (angle1 > angle2) {
return 1;
} else {
return Double.compare(p1.distance(minYPoint), p2.distance(minYPoint));
}
}
});
sortedPoints.add(0, minYPoint);
// 用栈来维护凸包上的点
Stack<Point> stack = new Stack<>();
stack.push(sortedPoints.get(0));
stack.push(sortedPoints.get(1));
for (int i = 2; i < sortedPoints.size(); i++) {
Point top = stack.pop();
while (ccw(stack.peek(), top, sortedPoints.get(i)) < 0) {
top = stack.pop();
}
stack.push(top);
stack.push(sortedPoints.get(i));
}
// 返回凸包上的点的列表
List<Point> convexHull = new ArrayList<>(stack);
convexHull.sort(new Comparator<Point>() {
@Override
public int compare(Point p1, Point p2) {
if (p1.x != p2.x) {
return Double.compare(p1.x, p2.x);
} else {
return Double.compare(p1.y, p2.y);
}
}
});
return convexHull;
}
private static int ccw(Point p1, Point p2, Point p3) {
double area2 = (p2.x - p1.x) * (p3.y - p1.y) - (p2.y - p1.y) * (p3.x - p1.x);
if (area2 > 0) {
return 1;
} else if (area2 < 0) {
return -1;
} else {
return 0;
}
}
public static class Point {
public final double x;
public final double y;
public Point(double x, double y) {
this.x = x;
this.y = y;
}
public double distance(Point other) {
double dx = this.x - other.x;
double dy = this.y - other.y;
return Math.sqrt(dx * dx + dy * dy);
}
@Override
public String toString() {
return "(" + x + ", " + y + ")";
}
}
}
```
使用方法:
```java
List<ConvexHull.Point> points = new ArrayList<>();
points.add(new ConvexHull.Point(0, 0));
points.add(new ConvexHull.Point(1, 1));
points.add(new ConvexHull.Point(2, 2));
points.add(new ConvexHull.Point(1, 2));
points.add(new ConvexHull.Point(2, 1));
List<ConvexHull.Point> convexHull = ConvexHull.getConvexHull(points);
System.out.println(convexHull);
// 输出:[(0.0, 0.0), (2.0, 2.0), (2.0, 1.0), (1.0, 2.0)]
```
该工具类中的 `Point` 类表示一个二维坐标点,`getConvexHull` 方法接受一个 `List<Point>` 类型的点集,返回凸包上的点的列表。
相关推荐
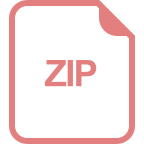
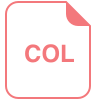













