private void executeUpdate(String sql) { try { Class.forName(driver); Connection con = DriverManager.getConnection(url); Statement cmd = con.createStatement(); cmd.executeUpdate(sql); con.close(); } catch (Exception ex) { ex.printStackTrace(); } }
时间: 2024-04-03 17:34:57 浏览: 97
这是一个Java方法,用于执行数据库更新操作。该方法接受一个SQL语句作为参数,然后使用JDBC连接到数据库并执行该语句。具体来说,它会加载指定的数据库驱动程序(使用Class.forName方法),然后使用DriverManager.getConnection方法连接到指定的数据库,创建一个Statement对象来执行SQL语句,最后关闭连接。请注意,该方法没有对SQL语句的有效性进行检查,因此需要确保传递给它的SQL语句是正确的。
相关问题
详细注释代码 public void del_cource (String id) throws SQLException, ClassNotFoundException { Class.forName("com.mysql.jdbc.Driver"); Connection connection = DriverManager.getConnection("jdbc:mysql://" + host + ":3306/db_stu_score", "root", ""); String sql = "DELETE from tb_cource where id = ?; "; PreparedStatement statement = connection.prepareStatement(sql); statement.setString(1, id); statement.executeUpdate(); statement.close(); connection.close(); }
这段代码是一个删除数据的方法,用于从数据库中删除指定id的课程记录。下面是对代码的详细注释:
```java
public void del_cource(String id) throws SQLException, ClassNotFoundException {
// 加载MySQL数据库驱动,这一步是必须的
Class.forName("com.mysql.jdbc.Driver");
// 连接到数据库
Connection connection = DriverManager.getConnection("jdbc:mysql://" + host + ":3306/db_stu_score", "root", "");
// SQL语句,用于删除指定id的课程记录
String sql = "DELETE from tb_cource where id = ?; ";
// 创建PreparedStatement对象,用于执行SQL语句
PreparedStatement statement = connection.prepareStatement(sql);
// 设置SQL语句中的参数,这里是id
statement.setString(1, id);
// 执行SQL语句
statement.executeUpdate();
// 关闭PreparedStatement对象
statement.close();
// 关闭数据库连接
connection.close();
}
```
这段代码中的主要步骤包括:
1. 加载MySQL数据库驱动;
2. 连接到数据库(这里的`host`是数据库的主机名,`db_stu_score`是数据库名,`root`是数据库用户名,空字符串是密码,如果有密码需要填写具体密码);
3. 构建SQL语句,用于删除指定id的课程记录;
4. 创建PreparedStatement对象,用于执行SQL语句;
5. 设置SQL语句中的参数,这里是id;
6. 执行SQL语句;
7. 关闭PreparedStatement对象;
8. 关闭数据库连接。
package db; import java.io.IOException; import java.io.InputStream; import java.sql.Connection; import java.sql.DriverManager; import java.sql.ResultSet; import java.sql.SQLException; import java.sql.Statement; import java.util.Properties; public class DBCon { Connection con = null; Statement st = null; ResultSet rs = null; String driver=null; String url = null; String username = null; String password = null; public Connection dbCon() { try { InputStream is=DBCon.class.getClassLoader().getResourceAsStream("db.properties"); Properties prop=new Properties(); try { prop.load(is); driver=prop.getProperty("driver"); url=prop.getProperty("url"); username=prop.getProperty("username"); password=prop.getProperty("password"); } catch (IOException e1) { e1.printStackTrace(); } Class.forName(driver); Class.forName("com.mysql.jdbc.Driver"); url = "jdbc:mysql:///sams?useUnicode=true&characterEncoding=utf8"; username = "root"; password = ""; try { con = DriverManager.getConnection(url, username, password); } catch (SQLException e) { e.printStackTrace(); } } catch (ClassNotFoundException e) { e.printStackTrace(); } return con; } /* * 增删改 */ public int query(String sql) { int rs = 0; con = dbCon(); try { st = con.createStatement(); rs = st.executeUpdate(sql); } catch (SQLException e) { close(); e.printStackTrace(); } return rs; } /* * 查 */ public ResultSet find(String sql) { try { con = dbCon(); st = con.createStatement(); rs = st.executeQuery(sql); } catch (SQLException e) { close(); e.printStackTrace(); } return rs; } /* * 关闭数据库 */ public void close() { try { if (rs != null) rs.close(); if (st != null) st.close(); if (con != null) con.close(); } catch (SQLException e) { e.printStackTrace(); } } }有错误
在 `dbCon` 方法中,你已经通过 `Properties` 对象获取了 `driver`、`url`、`username` 和 `password` 的值,但是之后又重新对这些变量进行了赋值,这会导致你在连接数据库时使用的是硬编码的值而不是从 `db.properties` 文件中读取的值。因此,需要删除这些重新赋值的语句:
```
Class.forName("com.mysql.jdbc.Driver");
url = "jdbc:mysql:///sams?useUnicode=true&characterEncoding=utf8";
username = "root";
password = "";
```
另外,你在 `find` 和 `query` 方法中都使用了 `con = dbCon();` 来获取连接,这会导致每次执行数据库操作都会重新获取一次连接,而不是复用已有的连接,这会导致性能问题。因此,应该在 `dbCon` 方法中增加一个判断,如果连接已经存在,则直接返回该连接,否则再新建连接。修改后的 `dbCon` 方法如下:
```
public Connection dbCon() {
if (con != null) {
return con;
}
try {
InputStream is=DBCon.class.getClassLoader().getResourceAsStream("db.properties");
Properties prop=new Properties();
try {
prop.load(is);
driver=prop.getProperty("driver");
url=prop.getProperty("url");
username=prop.getProperty("username");
password=prop.getProperty("password");
} catch (IOException e1) {
e1.printStackTrace();
}
Class.forName(driver);
con = DriverManager.getConnection(url, username, password);
} catch (ClassNotFoundException | SQLException e) {
e.printStackTrace();
}
return con;
}
```
阅读全文
相关推荐
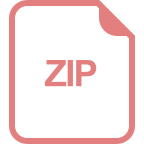














