C语言 求字符串长度,不用str cat函数
时间: 2024-01-21 12:16:18 浏览: 71
可以使用循环语句来求字符串长度,具体实现如下:
```c
#include <stdio.h>
int main() {
char str[] = "Hello, world!";
int len = 0;
while (str[len] != '\0') {
len++;
}
printf("The length of the string is %d\n", len);
return 0;
}
```
在这个程序中,我们首先定义了一个字符串 `str`,然后使用 `while` 循环来遍历字符串中的每一个字符,直到遇到字符串结束符 `\0` 为止。每遍历一个字符,就将字符串长度 `len` 加 1。最后输出求得的字符串长度即可。
相关问题
c语言:给字符串排序的函数怎么用
可以使用C语言中的库函数`qsort()`来对字符串进行排序。`qsort()`函数需要四个参数:待排序数组的首元素地址、待排序数组中元素的个数、每个元素的大小、比较函数的地址。比较函数用于指定两个元素的大小关系,如果第一个元素小于第二个元素,返回负整数;如果两个元素相等,返回0;如果第一个元素大于第二个元素,返回正整数。
下面是一个示例程序,演示如何使用`qsort()`函数对字符串数组进行排序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 比较函数,按字典序比较两个字符串的大小
int cmp(const void *a, const void *b) {
return strcmp(*(const char **)a, *(const char **)b);
}
int main() {
char *str[] = {"hello", "world", "apple", "banana", "cat"};
int len = sizeof(str) / sizeof(char *);
// 使用 qsort() 函数对字符串数组进行排序
qsort(str, len, sizeof(char *), cmp);
// 输出排序后的字符串数组
for (int i = 0; i < len; i++) {
printf("%s ", str[i]);
}
printf("\n");
return 0;
}
```
输出结果为:
```
apple banana cat hello world
```
在这个示例程序中,`cmp()`函数用于按字典序比较两个字符串的大小。`qsort()`函数将字符串数组`str`按照`cmp()`函数指定的比较方式进行排序。最后,使用循环遍历排序后的字符串数组,并输出每个字符串。
c语言单个字符串数组查找字符串
可以使用strcmp函数来对单个字符串数组进行查找。
例如,假设我们有一个字符串数组str_array,包含了多个字符串,我们想要查找其中的某个字符串target_str,可以使用以下代码:
```
#include <stdio.h>
#include <string.h>
int main() {
char str_array[10][20] = {"hello", "world", "foo", "bar", "apple", "banana", "cat", "dog", "sun", "moon"};
char target_str[20] = "banana";
int i, found = 0;
for (i = 0; i < 10; i++) {
if (strcmp(str_array[i], target_str) == 0) {
found = 1;
break;
}
}
if (found) {
printf("The target string \"%s\" is found at index %d.\n", target_str, i);
} else {
printf("The target string \"%s\" is not found in the array.\n", target_str);
}
return 0;
}
```
以上代码中,我们使用了一个双重循环来遍历字符串数组中的每个字符串,并使用strcmp函数逐个比较字符串,查找目标字符串。如果找到了目标字符串,我们设置found标记为1,并使用break语句跳出循环。最后,我们根据found标记的值输出查找结果。
阅读全文
相关推荐
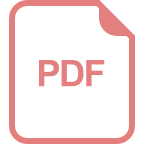
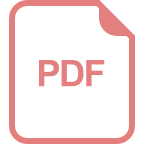
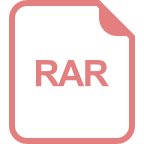













