请使用C++写一个动态链表,要求链表的结点由三种类,teacher,worker,leader三种类混合而成,根据数据输入的1,2,3来决定构建什么类,且这三种类都由基类employ构成,指针next也存在于基类中
时间: 2023-08-10 16:04:06 浏览: 98
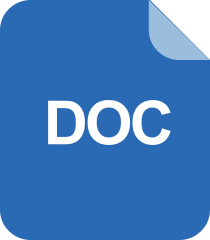
c++动态链表
以下是一个简单的动态链表代码,其中包含三种类:Teacher、Worker和Leader,都是由Employ类继承而来。
```c++
#include <iostream>
#include <string>
using namespace std;
class Employ {
public:
string name;
int age;
Employ* next; // 指向下一个节点的指针
Employ(string name, int age) {
this->name = name;
this->age = age;
next = NULL;
}
virtual void display() {
cout << "Name: " << name << ", Age: " << age << endl;
}
};
class Teacher : public Employ {
public:
string course;
Teacher(string name, int age, string course) : Employ(name, age) {
this->course = course;
}
void display() {
cout << "Name: " << name << ", Age: " << age << ", Course: " << course << endl;
}
};
class Worker : public Employ {
public:
string job;
Worker(string name, int age, string job) : Employ(name, age) {
this->job = job;
}
void display() {
cout << "Name: " << name << ", Age: " << age << ", Job: " << job << endl;
}
};
class Leader : public Employ {
public:
string department;
Leader(string name, int age, string department) : Employ(name, age) {
this->department = department;
}
void display() {
cout << "Name: " << name << ", Age: " << age << ", Department: " << department << endl;
}
};
int main() {
Employ* head = NULL;
while (true) {
int choice;
cout << "Please input 1 for Teacher, 2 for Worker, 3 for Leader, 0 to exit: ";
cin >> choice;
if (choice == 0) {
break;
}
string name;
int age;
cout << "Please input name: ";
cin >> name;
cout << "Please input age: ";
cin >> age;
Employ* emp;
switch (choice) {
case 1: {
string course;
cout << "Please input course: ";
cin >> course;
emp = new Teacher(name, age, course);
break;
}
case 2: {
string job;
cout << "Please input job: ";
cin >> job;
emp = new Worker(name, age, job);
break;
}
case 3: {
string department;
cout << "Please input department: ";
cin >> department;
emp = new Leader(name, age, department);
break;
}
default:
cout << "Invalid choice!" << endl;
continue;
}
if (head == NULL) {
head = emp;
} else {
Employ* current = head;
while (current->next != NULL) {
current = current->next;
}
current->next = emp;
}
}
Employ* current = head;
while (current != NULL) {
current->display();
current = current->next;
}
return 0;
}
```
阅读全文
相关推荐

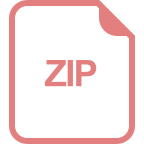
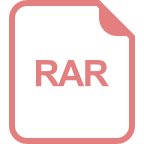
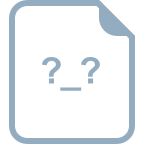
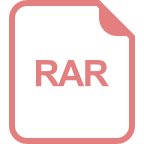
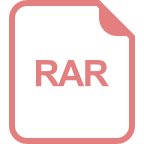
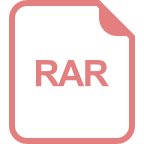
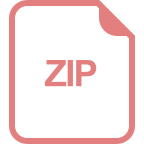
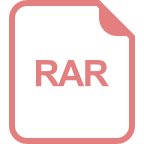
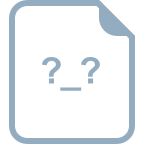
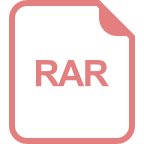
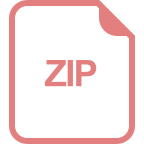
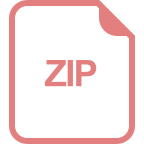
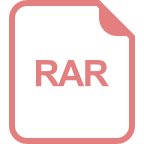