tensorflow识别物体并框出来
时间: 2023-12-05 17:05:44 浏览: 205
要使用TensorFlow识别物体并框出来,可以使用目标检测模型,如Faster R-CNN,SSD或YOLO等。这些模型可以识别图像中的物体,并用边界框框出它们。
以下是实现此功能的一些步骤:
1. 安装 TensorFlow Object Detection API
您需要安装TensorFlow Object Detection API,该API提供了训练和推断目标检测模型所需的工具和库。您可以在此处找到安装说明:https://github.com/tensorflow/models/blob/master/research/object_detection/g3doc/installation.md
2. 下载预训练模型
TensorFlow Object Detection API提供了一些预训练的目标检测模型,您可以从以下链接中选择一个模型并下载其预训练权重:https://github.com/tensorflow/models/blob/master/research/object_detection/g3doc/detection_model_zoo.md
3. 加载模型
使用TensorFlow Object Detection API加载预训练模型,并将其用于图像中的目标检测。您可以使用以下代码段加载模型:
```python
import tensorflow as tf
from object_detection.utils import label_map_util
from object_detection.utils import visualization_utils as vis_util
# Path to frozen detection graph. This is the actual model that is used for the object detection.
PATH_TO_CKPT = 'path/to/frozen_inference_graph.pb'
# List of the strings that is used to add correct label for each box.
PATH_TO_LABELS = 'path/to/label_map.pbtxt'
NUM_CLASSES = 90
# Load the frozen detection graph into memory
detection_graph = tf.Graph()
with detection_graph.as_default():
od_graph_def = tf.GraphDef()
with tf.gfile.GFile(PATH_TO_CKPT, 'rb') as fid:
serialized_graph = fid.read()
od_graph_def.ParseFromString(serialized_graph)
tf.import_graph_def(od_graph_def, name='')
# Load label map
label_map = label_map_util.load_labelmap(PATH_TO_LABELS)
categories = label_map_util.convert_label_map_to_categories(label_map, max_num_classes=NUM_CLASSES, use_display_name=True)
category_index = label_map_util.create_category_index(categories)
```
4. 运行推断
使用加载的模型运行推断,并绘制边界框以框出检测到的物体。您可以使用以下代码段运行推断:
```python
import numpy as np
import cv2
# Load image
image = cv2.imread('path/to/image.jpg')
# Define input and output tensors (i.e., image and bounding boxes)
image_tensor = detection_graph.get_tensor_by_name('image_tensor:0')
detection_boxes = detection_graph.get_tensor_by_name('detection_boxes:0')
detection_scores = detection_graph.get_tensor_by_name('detection_scores:0')
detection_classes = detection_graph.get_tensor_by_name('detection_classes:0')
num_detections = detection_graph.get_tensor_by_name('num_detections:0')
# Run inference
with detection_graph.as_default():
with tf.Session() as sess:
# Expand dimensions since the model expects images to have shape: [1, None, None, 3]
image_expanded = np.expand_dims(image, axis=0)
(boxes, scores, classes, num) = sess.run(
[detection_boxes, detection_scores, detection_classes, num_detections],
feed_dict={image_tensor: image_expanded})
# Visualize the results
vis_util.visualize_boxes_and_labels_on_image_array(
image,
np.squeeze(boxes),
np.squeeze(classes).astype(np.int32),
np.squeeze(scores),
category_index,
use_normalized_coordinates=True,
line_thickness=8)
# Display the resulting image
cv2.imshow('Object detection', cv2.resize(image, (800, 600)))
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这将在图像中绘制边界框,以框出检测到的物体。您可以根据需要调整绘制边界框的参数,例如线条宽度和颜色。
阅读全文
相关推荐
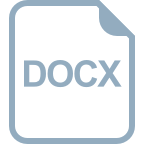
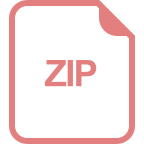















