写出iic的Verilog代码
时间: 2023-07-03 10:04:11 浏览: 162
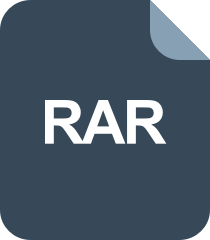
iic.rar_IIC scl sda_IIC.总线代码_iic代码Verilog_iic源码_verilog IIC
以下是一个简单的I2C总线控制器的Verilog代码:
```
module iic_controller(
input clk,
input rst_n,
input sda,
input scl,
output reg sda_out,
output reg sda_ack
);
// 定义I2C状态机的状态
localparam IDLE = 0, START = 1, WRITE = 2, READ = 3, STOP = 4;
// 定义I2C状态机的变量
reg [7:0] data_in;
reg [7:0] data_out;
reg [2:0] state_reg;
reg [2:0] state_next;
reg sda_dir;
// 初始化I2C状态机
always @(posedge clk or negedge rst_n) begin
if (!rst_n) begin
state_reg <= IDLE;
data_in <= 8'h00;
sda_out <= 1'b1;
sda_ack <= 1'b1;
sda_dir <= 1'b1;
end else begin
state_reg <= state_next;
data_in <= {data_in[6:0], sda};
end
end
// 定义I2C状态机的状态转换和输出逻辑
always @(*) begin
state_next = state_reg;
case (state_reg)
IDLE: begin
if (~sda & scl) begin
state_next = START;
end
end
START: begin
sda_out = 1'b0;
sda_dir = 1'b0;
if (scl) begin
state_next = WRITE;
end
end
WRITE: begin
sda_out = data_out[7];
sda_dir = 1'b0;
if (scl) begin
data_out <= {data_out[6:0], 1'b0};
if (data_out == 8'h00) begin
state_next = READ;
end
end
end
READ: begin
sda_out = 1'b1;
sda_dir = 1'b1;
if (scl) begin
if (data_in[7]) begin
sda_ack = 1'b0;
data_in <= {data_in[6:0], 1'b0};
end else begin
sda_ack = 1'b1;
state_next = STOP;
end
end
end
STOP: begin
sda_out = 1'b1;
sda_dir = 1'b0;
if (scl) begin
state_next = IDLE;
end
end
default: begin
state_next = IDLE;
end
endcase
end
// 设置SDA引脚的输入/输出方向
assign sda = (sda_dir) ? 1'bz : sda_out;
endmodule
```
这个I2C总线控制器实现了简单的主机模式,可以发送和接收8位数据。可以将其与外部I2C设备连接,并通过修改 `data_out` 和 `data_in` 来控制发送和接收的数据。
阅读全文
相关推荐
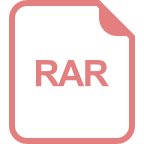
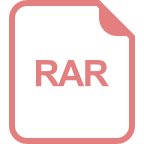
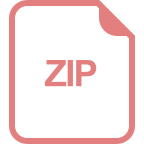



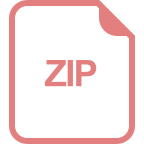
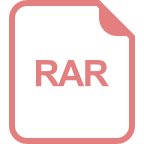
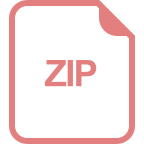
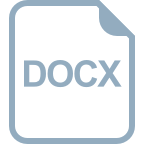
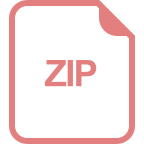
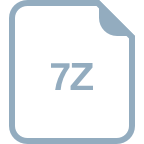
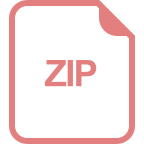
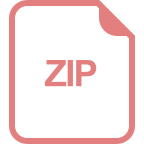