广告功能,广告信息包括广告名称,广告位置,开始日期,结束日期,广告链接,广告图片,请设计一个springboot项目,关联广告表,广告位置表,广告图片表,并详细展示增删查改广告信息的java代码
时间: 2023-07-31 17:05:21 浏览: 43
首先,我们需要设计数据库表结构。根据需求,我们需要设计三张表:广告表、广告位置表、广告图片表。
广告表(advertisement):
| 字段名 | 类型 | 说明 |
| ------------------ | ------------- | ------------ |
| id | bigint(20) | 主键ID |
| name | varchar(255) | 广告名称 |
| position_id | bigint(20) | 广告位置ID |
| start_date | datetime | 开始日期 |
| end_date | datetime | 结束日期 |
| link | varchar(255) | 广告链接 |
| image_id | bigint(20) | 广告图片ID |
| create_time | datetime | 创建时间 |
| update_time | datetime | 更新时间 |
广告位置表(ad_position):
| 字段名 | 类型 | 说明 |
| ------------------ | ------------- | ------------ |
| id | bigint(20) | 主键ID |
| name | varchar(255) | 位置名称 |
| create_time | datetime | 创建时间 |
| update_time | datetime | 更新时间 |
广告图片表(ad_image):
| 字段名 | 类型 | 说明 |
| ------------------ | ------------- | ------------ |
| id | bigint(20) | 主键ID |
| url | varchar(255) | 图片URL |
| create_time | datetime | 创建时间 |
| update_time | datetime | 更新时间 |
接下来,我们可以开始编写代码了。
首先,我们需要定义实体类Ad,包含上述广告表的字段:
```java
@Entity
@Table(name = "advertisement")
public class Ad {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@ManyToOne
@JoinColumn(name = "position_id")
private AdPosition position;
@Column(name = "start_date")
private LocalDateTime startDate;
@Column(name = "end_date")
private LocalDateTime endDate;
private String link;
@ManyToOne
@JoinColumn(name = "image_id")
private AdImage image;
@Column(name = "create_time")
private LocalDateTime createTime;
@Column(name = "update_time")
private LocalDateTime updateTime;
// getters and setters
}
```
接着定义实体类AdPosition和AdImage,分别对应广告位置表和广告图片表的字段:
```java
@Entity
@Table(name = "ad_position")
public class AdPosition {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@Column(name = "create_time")
private LocalDateTime createTime;
@Column(name = "update_time")
private LocalDateTime updateTime;
// getters and setters
}
@Entity
@Table(name = "ad_image")
public class AdImage {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String url;
@Column(name = "create_time")
private LocalDateTime createTime;
@Column(name = "update_time")
private LocalDateTime updateTime;
// getters and setters
}
```
然后,我们需要定义对应的Repository接口,用于数据访问:
```java
public interface AdRepository extends JpaRepository<Ad, Long> {
}
public interface AdPositionRepository extends JpaRepository<AdPosition, Long> {
}
public interface AdImageRepository extends JpaRepository<AdImage, Long> {
}
```
下一步,我们需要定义Service接口和实现类,用于业务逻辑处理:
```java
public interface AdService {
List<Ad> findAll();
Ad findById(Long id);
Ad save(Ad ad);
void deleteById(Long id);
}
@Service
@Transactional
public class AdServiceImpl implements AdService {
@Autowired
private AdRepository adRepository;
@Override
public List<Ad> findAll() {
return adRepository.findAll();
}
@Override
public Ad findById(Long id) {
return adRepository.findById(id).orElse(null);
}
@Override
public Ad save(Ad ad) {
return adRepository.save(ad);
}
@Override
public void deleteById(Long id) {
adRepository.deleteById(id);
}
}
```
接着,我们需要定义Controller类,用于接收请求和返回响应:
```java
@RestController
@RequestMapping("/ads")
public class AdController {
@Autowired
private AdService adService;
@GetMapping
public List<Ad> findAll() {
return adService.findAll();
}
@GetMapping("/{id}")
public Ad findById(@PathVariable Long id) {
return adService.findById(id);
}
@PostMapping
public Ad save(@RequestBody Ad ad) {
return adService.save(ad);
}
@PutMapping("/{id}")
public Ad update(@PathVariable Long id, @RequestBody Ad ad) {
Ad oldAd = adService.findById(id);
if (oldAd == null) {
throw new RuntimeException("Ad not found");
}
oldAd.setName(ad.getName());
oldAd.setPosition(ad.getPosition());
oldAd.setStartDate(ad.getStartDate());
oldAd.setEndDate(ad.getEndDate());
oldAd.setLink(ad.getLink());
oldAd.setImage(ad.getImage());
return adService.save(oldAd);
}
@DeleteMapping("/{id}")
public void deleteById(@PathVariable Long id) {
adService.deleteById(id);
}
}
```
最后,我们需要配置数据库连接信息和启动类:
```java
@SpringBootApplication
@EnableJpaRepositories
@EntityScan
public class AdApplication {
public static void main(String[] args) {
SpringApplication.run(AdApplication.class, args);
}
}
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
spring.datasource.url=jdbc:mysql://localhost:3306/ad?useUnicode=true&characterEncoding=utf8&useSSL=false
spring.datasource.username=root
spring.datasource.password=root
spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.MySQL5InnoDBDialect
spring.jpa.hibernate.ddl-auto=update
```
现在,我们就完成了广告信息的增删查改功能的代码实现。
相关推荐
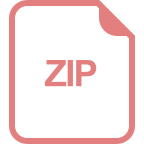
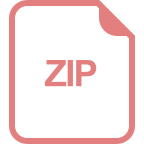
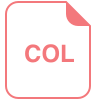
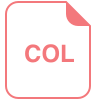
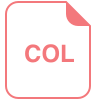
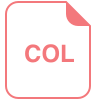
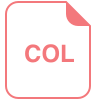









