把一个字符串中所有出现的大写字母都替换成小写字母,同时把小写字母替换成大写字母。
时间: 2023-09-08 11:05:17 浏览: 210
### 回答1:
可以使用Python实现:
```python
s = "HeLLo WoRLd"
s = ''.join([i.lower() if i.isupper() else i.upper() for i in s])
print(s)
```
输出结果为:
```
hEllO wOrlD
```
### 回答2:
要实现将一个字符串中的大写字母替换为小写字母,同时将小写字母替换为大写字母,可以使用循环遍历字符串,对每个字符进行判断及替换操作。以下是实现的相关代码:
```python
def convert_case(string):
result = ""
for char in string:
if char.isupper(): # 判断字符是否为大写字母
result += char.lower() # 大写字母替换为小写字母
elif char.islower(): # 判断字符是否为小写字母
result += char.upper() # 小写字母替换为大写字母
else:
result += char # 非字母字符则保持不变
return result
# 测试示例
input_string = "Hello World!"
output_string = convert_case(input_string)
print(output_string)
```
输出结果为:"hELLO wORLD!",即将原字符串中的大写字母全部替换为小写字母,同时将小写字母全部替换为大写字母。上述代码中使用了`isupper()`方法和`islower()`方法来判断字符是否为大写字母和小写字母,再使用`lower()`方法和`upper()`方法来进行大小写替换。最后得到替换后的结果字符串。
### 回答3:
将一个字符串中的所有大写字母替换为小写字母,小写字母替换为大写字母的方法是通过循环遍历字符串中的每个字符,然后判断字符的ASCII码值是否在大写或小写字母的ASCII码范围内,如果是,就相应地将其转换为另一种大小写形式。
步骤如下:
1. 定义一个空字符串作为结果变量,用于存储转换后的字符串。
2. 使用for循环遍历字符串中的每个字符。
3. 在循环中,使用ord()函数获取当前字符的ASCII码值,然后通过判断这个值是否在大写或小写字母的ASCII码范围内来确定当前字符的大小写形式。
4. 如果ASCII码值在大写字母范围内(65到90),则使用chr()函数将其转换为小写字母形式,并将转换后的字符添加到结果字符串中。
5. 如果ASCII码值在小写字母范围内(97到122),则使用chr()函数将其转换为大写字母形式,并将转换后的字符添加到结果字符串中。
6. 如果ASCII码值既不在大写字母范围内,也不在小写字母范围内,则说明该字符不是字母,直接将其添加到结果字符串中。
7. 循环结束后,返回结果字符串。
以下是一个示例代码:
```python
def convert_case(string):
result = ""
for char in string:
ascii_value = ord(char)
if 65 <= ascii_value <= 90:
converted_char = chr(ascii_value + 32)
elif 97 <= ascii_value <= 122:
converted_char = chr(ascii_value - 32)
else:
converted_char = char
result += converted_char
return result
```
使用示例:
```python
string = "HelloWorld!"
converted_string = convert_case(string)
print(converted_string)
```
输出结果为:
```
hELLOwORLD!
```
即将所有大写字母替换为小写字母,小写字母替换为大写字母的结果。
阅读全文
相关推荐
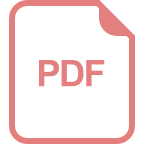
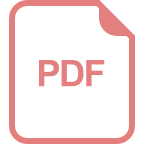


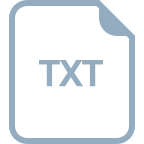
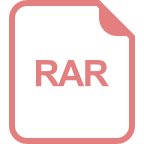
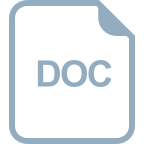
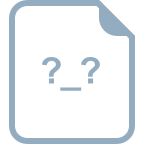
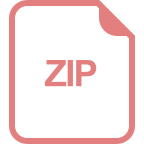
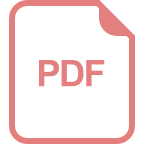
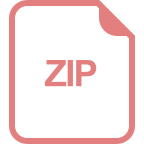
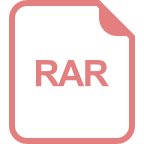
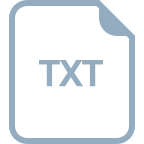
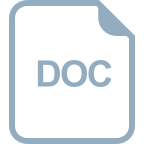
