如何实现360度视频生成全景图拼接 请给出解释和代码
时间: 2023-06-15 07:05:40 浏览: 150
360度视频生成全景图拼接的方法一般包括以下步骤:
1. 提取视频帧:从360度视频中提取出每一帧图像。
2. 图像校正:将提取出的图像进行校正,使其能够正确地贴在全景图上。
3. 图像拼接:将校正后的图像拼接成全景图。
以下是一个基于Python和OpenCV的360度视频生成全景图拼接的代码示例:
```python
import cv2
import numpy as np
# 读取视频文件
cap = cv2.VideoCapture('360video.mp4')
# 定义横向和纵向的视角范围
h_fov = 360
v_fov = 180
# 定义输出图像的大小
out_width = 4000
out_height = int(out_width / h_fov * v_fov)
# 创建输出图像
panorama = np.zeros((out_height, out_width, 3), dtype=np.uint8)
# 定义相机的投影矩阵
focal_length = out_width / (2 * np.tan(h_fov * np.pi / 360))
camera_matrix = np.array([[focal_length, 0, out_width / 2], [0, focal_length, out_height / 2], [0, 0, 1]])
# 循环读取视频帧
while cap.isOpened():
ret, frame = cap.read()
if not ret:
break
# 对图像进行校正
map_x, map_y = cv2.initUndistortRectifyMap(camera_matrix, None, None, camera_matrix, (frame.shape[1], frame.shape[0]), cv2.CV_32FC1)
frame = cv2.remap(frame, map_x, map_y, cv2.INTER_LINEAR)
# 将校正后的图像拼接到全景图上
x_offset = int((frame.shape[1] - h_fov) / 2)
y_offset = int((frame.shape[0] - v_fov) / 2)
panorama[y_offset:y_offset+v_fov, x_offset:x_offset+h_fov, :] = frame[:v_fov, :h_fov, :]
# 保存全景图
cv2.imwrite('panorama.jpg', panorama)
```
代码中使用了OpenCV的`cv2.initUndistortRectifyMap`和`cv2.remap`函数对图像进行校正,并将校正后的图像拼接到全景图上。其中,相机的投影矩阵通过计算得到,具体的计算方法可以参考相关文献。最后,保存拼接后的全景图。
相关推荐
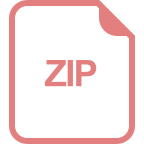
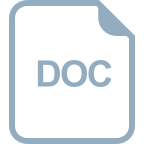
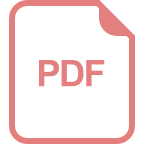
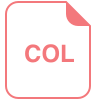













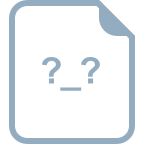