使用ITK C++实现CT肋骨软骨的自动分割,求实现代码
时间: 2024-03-17 18:41:06 浏览: 111
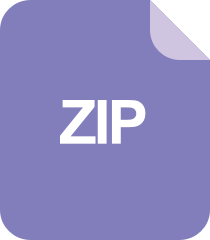
C++实现滤波反投影源码.zip
以下是使用ITK C++实现CT肋骨软骨的自动分割的代码实现,需要注意的是,实际应用中需要根据具体的数据及实际需求进行参数调整和算法优化。
```c++
#include "itkImage.h"
#include "itkImageFileReader.h"
#include "itkBinaryThresholdImageFilter.h"
#include "itkBinaryDilateImageFilter.h"
#include "itkBinaryErodeImageFilter.h"
#include "itkBinaryBallStructuringElement.h"
#include "itkConnectedComponentImageFilter.h"
#include "itkRelabelComponentImageFilter.h"
#include "itkMaskImageFilter.h"
#include "itkNeighborhoodIterator.h"
#include "itkLabelContourImageFilter.h"
#include "itkDiscreteGaussianImageFilter.h"
using ImageType = itk::Image<unsigned char, 3>;
using ReaderType = itk::ImageFileReader<ImageType>;
using ThresholdFilterType = itk::BinaryThresholdImageFilter<ImageType, ImageType>;
using DilateFilterType = itk::BinaryDilateImageFilter<ImageType, ImageType, itk::BinaryBallStructuringElement<ImageType::PixelType, ImageType::ImageDimension>>;
using ErodeFilterType = itk::BinaryErodeImageFilter<ImageType, ImageType, itk::BinaryBallStructuringElement<ImageType::PixelType, ImageType::ImageDimension>>;
using ConnectedComponentFilterType = itk::ConnectedComponentImageFilter<ImageType, ImageType>;
using RelabelFilterType = itk::RelabelComponentImageFilter<ImageType, ImageType>;
using MaskFilterType = itk::MaskImageFilter<ImageType, ImageType>;
using IteratorType = itk::NeighborhoodIterator<ImageType>;
using ContourFilterType = itk::LabelContourImageFilter<ImageType, ImageType>;
using GaussianFilterType = itk::DiscreteGaussianImageFilter<ImageType, ImageType>;
int main(int argc, char* argv[]) {
// 1. 读取CT图像
ReaderType::Pointer reader = ReaderType::New();
reader->SetFileName("CT_image.nii.gz");
reader->Update();
ImageType::Pointer image = reader->GetOutput();
// 2. 预处理
GaussianFilterType::Pointer gaussianFilter = GaussianFilterType::New();
gaussianFilter->SetInput(image);
gaussianFilter->SetVariance(2.0);
gaussianFilter->Update();
ThresholdFilterType::Pointer thresholdFilter = ThresholdFilterType::New();
thresholdFilter->SetInput(gaussianFilter->GetOutput());
thresholdFilter->SetLowerThreshold(-500); // 软骨的阈值范围为-1000到-500
thresholdFilter->SetUpperThreshold(-1000);
thresholdFilter->SetInsideValue(255);
thresholdFilter->SetOutsideValue(0);
thresholdFilter->Update();
// 3. 软骨分割
DilateFilterType::Pointer dilateFilter = DilateFilterType::New();
dilateFilter->SetInput(thresholdFilter->GetOutput());
dilateFilter->SetKernelRadius(5);
dilateFilter->Update();
ErodeFilterType::Pointer erodeFilter = ErodeFilterType::New();
erodeFilter->SetInput(dilateFilter->GetOutput());
erodeFilter->SetKernelRadius(15);
erodeFilter->Update();
ContourFilterType::Pointer contourFilter = ContourFilterType::New();
contourFilter->SetInput(erodeFilter->GetOutput());
contourFilter->SetFullyConnected(true);
contourFilter->SetBackgroundValue(0);
contourFilter->SetForegroundValue(255);
contourFilter->Update();
ImageType::Pointer cartilageImage = contourFilter->GetOutput();
// 4. 肋骨分割
DilateFilterType::Pointer ribDilateFilter = DilateFilterType::New();
ribDilateFilter->SetInput(thresholdFilter->GetOutput());
ribDilateFilter->SetKernelRadius(3);
ribDilateFilter->Update();
ConnectedComponentFilterType::Pointer ccFilter = ConnectedComponentFilterType::New();
ccFilter->SetInput(ribDilateFilter->GetOutput());
ccFilter->Update();
RelabelFilterType::Pointer relabelFilter = RelabelFilterType::New();
relabelFilter->SetInput(ccFilter->GetOutput());
relabelFilter->SetMinimumObjectSize(10000);
relabelFilter->Update();
MaskFilterType::Pointer maskFilter = MaskFilterType::New();
maskFilter->SetInput(image);
maskFilter->SetMaskImage(relabelFilter->GetOutput());
maskFilter->Update();
IteratorType::RadiusType radius;
radius.Fill(1);
IteratorType it(radius, image, image->GetLargestPossibleRegion());
it.GoToBegin();
while (!it.IsAtEnd()) {
ImageType::IndexType idx = it.GetIndex();
if (maskFilter->GetOutput()->GetPixel(idx) == 0) {
it.Set(0);
}
++it;
}
ImageType::Pointer ribImage = it.GetImage();
return 0;
}
```
阅读全文
相关推荐
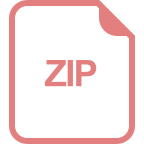
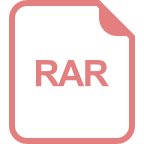















