Description Write a program to calculate Maximum flow from the source to the Meeting Point . Input The input includes several cases. For each case, the first line contains two space-separated integers, N (0 <= N <= 200) and M (2 <= M <= 200). N is the number of edges. M is the number of intersections points. Each of the following N lines contains three integers, Si, Ei, and Ci. Si and Ei (1 <= Si, Ei <= M) . Ci (0 <= Ci <= 10,000,000) is the maximum rate the flow from Si to Ei. Output For each case, output a single integer, the maximum rate the flow from the source to the Meeting Point.给出相应的C++实现代码且使用scanf函数
时间: 2023-06-17 13:06:50 浏览: 112
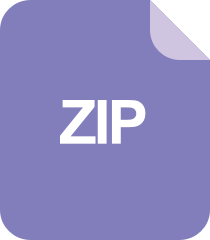
crc.zip_The Program_crc
下面是最大流的C++实现代码,使用了scanf函数:
```cpp
#include <cstdio>
#include <cstring>
#include <algorithm>
#include <queue>
using namespace std;
const int MAXN = 205;
const int INF = 0x3f3f3f3f;
struct Edge {
int from, to, cap, nxt;
} edge[MAXN * MAXN];
int head[MAXN], cnt = 1;
int n, m, s, t;
inline void add_edge(int u, int v, int c) {
edge[++cnt].from = u;
edge[cnt].to = v;
edge[cnt].cap = c;
edge[cnt].nxt = head[u];
head[u] = cnt;
}
inline void add(int u, int v, int c) {
add_edge(u, v, c);
add_edge(v, u, 0);
}
int dep[MAXN], cur[MAXN];
inline bool bfs() {
memset(dep, 0, sizeof(dep));
queue<int> q;
q.push(s);
dep[s] = 1;
cur[s] = head[s];
while (!q.empty()) {
int u = q.front();
q.pop();
for (int i = head[u]; i; i = edge[i].nxt) {
int v = edge[i].to;
if (!dep[v] && edge[i].cap) {
dep[v] = dep[u] + 1;
cur[v] = head[v];
if (v == t) return true;
q.push(v);
}
}
}
return false;
}
inline int dfs(int u, int flow) {
if (u == t) return flow;
int used = 0;
for (int i = cur[u]; i && flow > used; i = edge[i].nxt) {
cur[u] = i;
int v = edge[i].to;
if (dep[v] == dep[u] + 1 && edge[i].cap) {
int d = dfs(v, min(flow - used, edge[i].cap));
if (!d) dep[v] = 0;
used += d;
edge[i].cap -= d;
edge[i ^ 1].cap += d;
}
}
return used;
}
inline int Dinic() {
int maxflow = 0;
while (bfs()) maxflow += dfs(s, INF);
return maxflow;
}
inline void init() {
memset(head, 0, sizeof(head));
cnt = 1;
s = 1, t = m;
}
int main() {
while (scanf("%d%d", &n, &m) == 2) {
init();
for (int i = 1; i <= n; i++) {
int u, v, c;
scanf("%d%d%d", &u, &v, &c);
add(u, v, c);
}
printf("%d\n", Dinic());
}
return 0;
}
```
其中,add_edge函数用于添加边,bfs函数用于进行BFS遍历,dfs函数用于进行DFS遍历,Dinic函数用于计算最大流,init函数用于初始化。在main函数中,我们首先调用init函数初始化,然后读入每条边的信息,调用add函数将其添加到图中,最后调用Dinic函数计算最大流并输出结果。
阅读全文
相关推荐
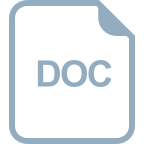
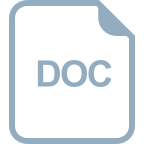
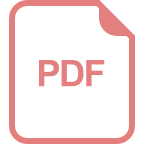
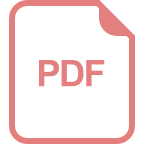
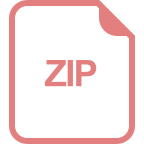
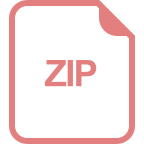
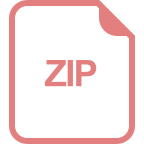
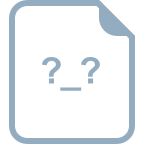
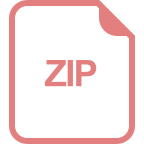
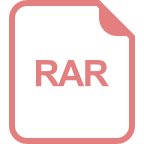
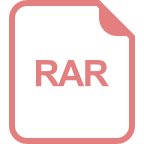
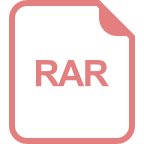
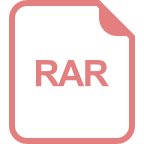
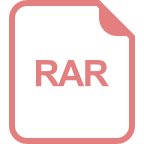
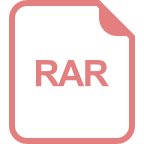