JAVA存取PostgreSQL二进制类型bytea
时间: 2024-02-23 19:01:12 浏览: 23
在Java中,可以使用JDBC驱动程序来存取PostgreSQL中的二进制类型bytea。下面是一个简单的示例代码:
```java
import java.sql.*;
public class BinaryDataExample {
public static void main(String[] args) {
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
// Load the PostgreSQL JDBC driver
Class.forName("org.postgresql.Driver");
// Connect to the database
conn = DriverManager.getConnection("jdbc:postgresql://localhost/mydatabase", "myusername", "mypassword");
// Insert binary data into the database
pstmt = conn.prepareStatement("INSERT INTO mytable (mybinarydata) VALUES (?)");
byte[] binaryData = {1, 2, 3, 4, 5};
ByteArrayInputStream bais = new ByteArrayInputStream(binaryData);
pstmt.setBinaryStream(1, bais, binaryData.length);
pstmt.executeUpdate();
// Retrieve binary data from the database
pstmt = conn.prepareStatement("SELECT mybinarydata FROM mytable WHERE id = ?");
pstmt.setInt(1, 1);
rs = pstmt.executeQuery();
if (rs.next()) {
// Read binary data from the result set
InputStream is = rs.getBinaryStream("mybinarydata");
byte[] buffer = new byte[1024];
int bytesRead = 0;
while ((bytesRead = is.read(buffer)) != -1) {
// Process binary data
}
}
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (rs != null) {
rs.close();
}
if (pstmt != null) {
pstmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
```
上面的代码演示了如何将一个字节数组插入到PostgreSQL数据库中的bytea字段中,并从数据库中检索出它。在插入时,我们使用了PreparedStatement的setBinaryStream方法,该方法允许我们将一个字节数组作为InputStream插入到数据库中。在检索时,我们使用了ResultSet的getBinaryStream方法,该方法返回一个InputStream对象,我们可以使用它来读取存储在数据库中的二进制数据。
相关推荐
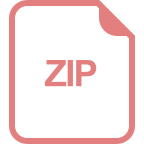
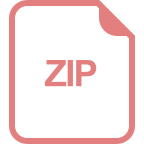














