ajax.send({})
时间: 2023-07-20 21:42:38 浏览: 44
`ajax.send({})` 并不是 AJAX 的正确语法。正确的 AJAX 请求通常使用 `XMLHttpRequest` 对象来发送请求,`send` 方法接收一个参数,即要发送的请求数据。常见的语法如下:
```
var xmlhttp = new XMLHttpRequest(); // 创建一个 XMLHttpRequest 对象
xmlhttp.onreadystatechange = function() { // 监听状态变化事件
if (this.readyState == 4 && this.status == 200) { // 当 readyState 为 4,status 为 200 时表示响应已就绪
console.log(this.responseText); // 打印响应内容
}
};
xmlhttp.open("GET", "example.php?name=value", true); // 指定请求方法、URL 和是否为异步请求
xmlhttp.send(); // 发送请求
```
在这个例子中,我们使用 `GET` 请求方式来向 `example.php` 发送请求,并且带有一个名为 `name` 的参数,值为 `value`。在发送请求后,我们监听 `onreadystatechange` 事件,当事件触发时,判断 `readyState` 和 `status` 是否符合预期,如果符合说明响应已就绪,我们可以通过 `responseText` 属性获取响应内容。
相关问题
Ember.js中的 ajax.send用法
在 Ember.js 中,`ajax.send()` 并不是官方推荐的方式来发送 AJAX 请求,而是底层的私有 API。官方推荐的方式是使用 `Ember.$.ajax()` 或 `Ember.ajax()`。
如果你非常确定要使用 `ajax.send()` 发送请求,可以按照以下方式使用:
```
import Ember from 'ember';
export default Ember.Route.extend({
actions: {
submitForm() {
const data = {
username: 'john'
};
Ember.$.ajax({
url: '/api/users',
type: 'POST',
data: JSON.stringify(data)
}).then(function(response) {
console.log(response);
}).catch(function(error) {
console.log(error);
});
}
},
setupController(controller, model) {
this._super(controller, model);
const ajax = Ember.$.ajax({
url: '/api/users',
type: 'GET'
});
ajax.then(function(response) {
console.log(response);
});
ajax.catch(function(error) {
console.log(error);
});
ajax.send(); // 发送请求
}
});
```
在这个例子中,我们在 `setupController` 钩子函数中使用 `Ember.$.ajax()` 创建一个 AJAX 请求对象 `ajax`,然后使用 `ajax.send()` 方法发送请求。在 `submitForm` 钩子函数中,我们使用 `Ember.$.ajax()` 直接发送一个 POST 请求。
需要注意的是,使用 `ajax.send()` 发送请求时,必须先创建一个 AJAX 请求对象,然后在对象上调用 `send()` 方法。此外,在 Ember.js 中,不建议直接使用底层的私有 API,因为它可能会在未来的版本中发生变化。如果可能的话,应该尽量使用官方推荐的方式发送 AJAX 请求。
查找错误点<script> var mcText = document.querySelector(`.mcText`) var accountedText = document.querySelector('.accountedText') var passwordText = document.querySelector('.passwordText') var registerDiv = document.querySelector('.registerDiv') var isAgree = false; //注册按钮的点击事件 registerDiv.onclick = function () { if(isAgree == true){ // history.back() var ajax = new XMLHttpRequest() var string = "mc="+mcText.value+"username="+accoundedText.value+'&password='+passwordText.value; ajax.open('get','http://localhost:8080/registUser?'+string) ajax.send() } else { alert("请输入名称账号密码") } } ajax.onload = function (){ console.log(ajax.responseText); } //监听这三个输入框失去焦点 mcText.onblur = function (){ isAgree = isAgreeLogin(); } accountedText.onblur = function (){ isAgree = isAgreeLogin(); } passwordText.onblur = function (){ isAgree = isAgreeLogin(); } //在失去焦点的时候,判断三个输入框是否都有值了,如果有则高亮显示,如果无则不亮 function isAgreeLogin() { if(mcText.value !='' && accountedText.value != ''&& passwordText.value != ''){ registerDiv.style.backgroundColor = "#648ceb" registerDiv.style.boxShadow = "2px 2px 2px #9eb8f6" return true } else { return false } } </script>
您的代码中应该把 `var ajax = new XMLHttpRequest()` 放到 `registerDiv.onclick` 的函数体内部,否则会造成 ajax 未定义的错误。
正确的代码应该是这样的:
```
var mcText = document.querySelector(`.mcText`);
var accountedText = document.querySelector('.accountedText');
var passwordText = document.querySelector('.passwordText');
var registerDiv = document.querySelector('.registerDiv');
var isAgree = false;
registerDiv.onclick = function () {
if (isAgree == true) {
var ajax = new XMLHttpRequest(); // 将此行代码放到函数体内部
var string = "mc=" + mcText.value + "username=" + accountedText.value + '&password=' + passwordText.value;
ajax.open('get', 'http://localhost:8080/registUser?' + string);
ajax.send();
} else {
alert("请输入名称账号密码");
}
}
ajax.onload = function () {
console.log(ajax.responseText);
}
mcText.onblur = function () {
isAgree = isAgreeLogin();
}
accountedText.onblur = function () {
isAgree = isAgreeLogin();
}
passwordText.onblur = function () {
isAgree = isAgreeLogin();
}
function isAgreeLogin() {
if (mcText.value != '' && accountedText.value != '' && passwordText.value != '') {
registerDiv.style.backgroundColor = "#648ceb";
registerDiv.style.boxShadow = "2px 2px 2px #9eb8f6";
return true;
} else {
return false;
}
}
```
请注意,这只是一种可能的解决方案。如果问题仍然存在,请提供更多上下文信息和完整的代码,以便我更好地帮助您。
相关推荐
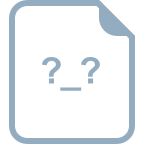
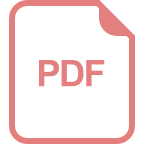
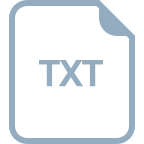












