写一个安卓httppost请求
时间: 2023-09-08 18:05:17 浏览: 127
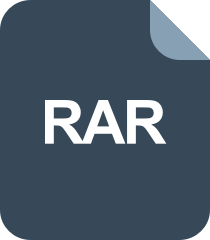
android的post请求

### 回答1:
我可以为您提供一个简单的安卓HTTP POST请求的示例: // 创建一个URL对象
URL url = new URL("http://example.com/post"); // 打开连接
HttpURLConnection con = (HttpURLConnection) url.openConnection(); // 设置请求方式
con.setRequestMethod("POST"); // 添加请求头
con.setRequestProperty("User-Agent", "Mozilla/5.0"); // 发送POST请求必须设置如下两行
con.setDoOutput(true);
con.setDoInput(true); // 获取URLConnection对象对应的输出流
PrintWriter out = new PrintWriter(con.getOutputStream()); // 发送请求参数
out.print("param1=value1¶m2=value2"); // flush输出流的缓冲
out.flush(); // 定义BufferedReader输入流来读取URL的响应
BufferedReader in = new BufferedReader(new InputStreamReader(con.getInputStream()));
String line;
while ((line = in.readLine()) != null) {
// 处理响应内容
}
### 回答2:
在安卓平台上发送一个HttpPost请求非常简单,可以按照以下步骤进行:
1. 首先,确保你已经添加了网络权限到AndroidManifest.xml文件中:
```xml
<uses-permission android:name="android.permission.INTERNET" />
```
2. 在你的Activity或者Fragment中创建一个Http请求方法,例如:
```java
private void sendPostRequest() {
// 创建HttpClient实例
HttpClient httpClient = new DefaultHttpClient();
// 创建HttpPost对象,并指定请求的URL地址
HttpPost httpPost = new HttpPost("http://example.com/api");
try {
// 创建请求参数
List<NameValuePair> params = new ArrayList<>();
params.add(new BasicNameValuePair("param1", "value1")); // 添加参数1
// 将请求参数进行编码
httpPost.setEntity(new UrlEncodedFormEntity(params));
// 执行Http请求
HttpResponse response = httpClient.execute(httpPost);
// 获取响应结果
InputStream inputStream = response.getEntity().getContent();
BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(inputStream));
String line;
StringBuilder result = new StringBuilder();
while ((line = bufferedReader.readLine()) != null) {
result.append(line);
}
// 处理响应结果
// ...
// 关闭输入流和HttpClient实例
inputStream.close();
httpClient.getConnectionManager().shutdown();
} catch (IOException e) {
e.printStackTrace();
}
}
```
3. 调用`sendPostRequest()`方法来发送HttpPost请求,例如在Activity的onCreate()方法中:
```java
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
sendPostRequest();
}
```
以上是一个简单的安卓平台中发送HttpPost请求的示例,你可以根据自己的需求对请求参数进行修改,以及根据响应结果进行处理。
### 回答3:
安卓中进行HTTP POST请求可以通过使用HttpURLConnection类来实现。下面是一个简单的示例代码:
```java
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.OutputStreamWriter;
import java.net.HttpURLConnection;
import java.net.URL;
public class HttpPostRequestExample {
public static void main(String[] args) {
HttpURLConnection connection = null;
try {
// 创建URL对象
URL url = new URL("http://example.com/api/endpoint");
// 创建HTTP连接
connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("POST");
connection.setRequestProperty("Content-Type", "application/json");
// 设置要发送的数据
String requestData = "{\"key\":\"value\"}";
// 启用输出流
connection.setDoOutput(true);
// 写入数据
OutputStreamWriter writer = new OutputStreamWriter(connection.getOutputStream());
writer.write(requestData);
writer.flush();
writer.close();
// 获取响应结果
BufferedReader reader = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String line;
StringBuilder response = new StringBuilder();
while ((line = reader.readLine()) != null) {
response.append(line);
}
reader.close();
// 输出响应结果
System.out.println(response.toString());
} catch (IOException e) {
e.printStackTrace();
} finally {
if (connection != null) {
connection.disconnect();
}
}
}
}
```
在上面的示例中,我们首先创建了一个URL对象,用于表示要发送请求的目标地址。然后,使用HttpURLConnection类来打开与该URL的连接。我们设置请求的方法为POST,并设置Content-Type为application/json,以便服务器可以正确解析请求数据。然后,我们启用了输出流,并将要发送的数据写入输出流中。最后,我们读取服务器的响应结果,并将其打印到控制台上。
请注意,这只是一个简单的示例,实际应用中可能还需要处理异常、验证服务端返回的状态码等。
阅读全文
相关推荐
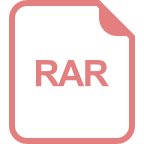
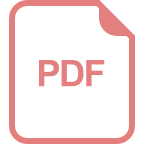
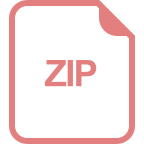
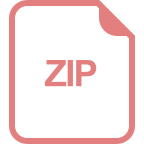
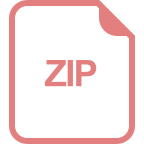
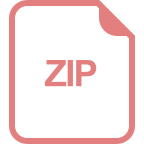
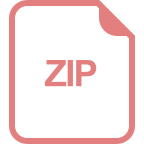
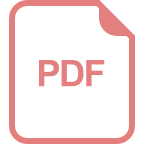
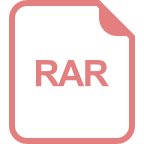
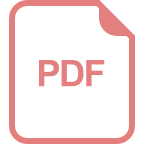
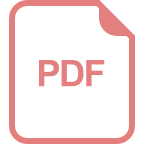
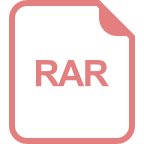
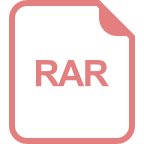
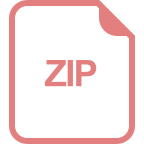
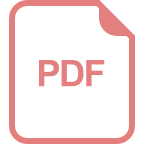