c51单片机温度传感器dht11精联讯屏幕显示
时间: 2023-09-25 16:15:39 浏览: 52
这是一个基于c51单片机和温度传感器dht11的温度监测系统,通过精联讯的屏幕显示实时温度数据。
硬件模块:
- c51单片机
- 温度传感器dht11
- 精联讯LCD屏幕
软件模块:
- C语言编程
- Keil C51开发环境
- DHT11驱动程序
- LCD屏幕驱动程序
实现流程:
1. 初始化温度传感器和LCD屏幕。
2. 循环读取温度传感器数据,计算得到实时温度。
3. 将实时温度数据显示在LCD屏幕上。
代码示例:
```
#include <reg52.h>
#include <intrins.h>
#define uchar unsigned char
#define uint unsigned int
sbit DHT11_DATA = P1^0; // 温度传感器数据引脚
sbit LCD_RS = P2^0; // LCD屏幕RS引脚
sbit LCD_RW = P2^1; // LCD屏幕RW引脚
sbit LCD_EN = P2^2; // LCD屏幕EN引脚
sbit LCD_BL = P2^3; // LCD屏幕背光引脚
uchar code Table[] = "Temperature:"; // LCD屏幕显示字符串
void delay_us(uint us)
{
while(us--)
{
_nop_();
_nop_();
}
}
void delay_ms(uint ms)
{
while(ms--)
{
delay_us(1000);
}
}
void LCD_Init()
{
LCD_BL = 1; // 开启背光
LCD_RS = 0;
LCD_RW = 0;
LCD_EN = 0;
delay_ms(15);
LCD_SendCmd(0x38); // 设置16*2显示模式
delay_ms(5);
LCD_SendCmd(0x38);
delay_ms(5);
LCD_SendCmd(0x38);
delay_ms(5);
LCD_SendCmd(0x0c); // 开启显示
delay_ms(5);
LCD_SendCmd(0x01); // 清屏
delay_ms(5);
}
void LCD_SendCmd(uchar cmd)
{
LCD_RS = 0;
LCD_RW = 0;
LCD_EN = 1;
P0 = cmd;
delay_us(5);
LCD_EN = 0;
}
void LCD_SendData(uchar dat)
{
LCD_RS = 1;
LCD_RW = 0;
LCD_EN = 1;
P0 = dat;
delay_us(5);
LCD_EN = 0;
}
void LCD_DisplayString(uchar x, uchar y, uchar *str)
{
uchar i = 0;
if(x < 16)
{
if(y == 0)
{
LCD_SendCmd(0x80 + x);
}
else
{
LCD_SendCmd(0x80 + 0x40 + x);
}
}
while(str[i] != '\0')
{
LCD_SendData(str[i++]);
}
}
uchar DHT11_ReadData()
{
uchar i, j, res;
for(i = 0; i < 8; i++)
{
DHT11_DATA = 0;
delay_us(18);
DHT11_DATA = 1;
delay_us(40);
res <<= 1;
if(DHT11_DATA)
{
res |= 1;
}
delay_us(40);
}
return res;
}
void DHT11_ReadTemp(uchar *temp)
{
uchar i, j, check_sum;
DHT11_DATA = 0;
delay_ms(20);
DHT11_DATA = 1;
delay_us(30);
if(DHT11_DATA)
{
LCD_DisplayString(0, 0, "DHT11 Error!");
return;
}
delay_us(80);
if(!DHT11_DATA)
{
LCD_DisplayString(0, 0, "DHT11 Error!");
return;
}
delay_us(80);
for(i = 0; i < 5; i++)
{
temp[i] = DHT11_ReadData();
}
check_sum = temp[0] + temp[1] + temp[2] + temp[3];
if(check_sum != temp[4])
{
LCD_DisplayString(0, 0, "DHT11 Error!");
return;
}
}
void main()
{
uchar temp[5];
LCD_Init();
while(1)
{
DHT11_ReadTemp(temp);
LCD_DisplayString(0, 0, Table);
LCD_DisplayString(0, 1, temp);
delay_ms(1000);
}
}
```
相关推荐
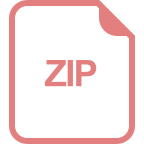














