C++将字符串转为数字
时间: 2024-04-28 10:21:49 浏览: 12
在 C++ 中将字符串转化为数字可以使用 `std::stoi()` 或 `std::stof()` 函数,具体使用哪个函数取决于字符串中包含的是整数还是浮点数。
例如,将字符串 `"123"` 转化为整数可以使用 `std::stoi("123")` 函数,结果为 `123`。而将字符串 `"3.14"` 转化为浮点数可以使用 `std::stof("3.14")` 函数,结果为 `3.14`。
需要注意的是,如果字符串中包含除数字外的其他字符,那么转化会失败并抛出异常。因此在使用 `std::stoi()` 或 `std::stof()` 函数前需要确保字符串只包含数字。另外,这两个函数还有一些可选参数,可以在转化时指定进制、舍入方式等参数。
相关问题
c++将字符串转换为数字
可以使用 `stoi` 函数将字符串转换为整数,或者使用 `stof` 函数将字符串转换为浮点数。示例如下:
```c++
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "123";
int num = stoi(str);
cout << num << endl;
string str2 = "3.14";
float fnum = stof(str2);
cout << fnum << endl;
return 0;
}
```
输出结果为:
```
123
3.14
```
C++将字符串转为数字的代码
以下是 C++ 中将字符串转化为数字的示例代码:
```
#include <iostream>
#include <string>
int main() {
std::string str_int = "123";
std::string str_float = "3.14";
int num_int = std::stoi(str_int);
float num_float = std::stof(str_float);
std::cout << "The integer value is " << num_int << std::endl;
std::cout << "The float value is " << num_float << std::endl;
return 0;
}
```
以上代码将字符串 `"123"` 转化为整数,字符串 `"3.14"` 转化为浮点数,并输出转化后的结果。需要注意的是,这里使用了 `<string>` 头文件中的 `std::string` 类型。
相关推荐
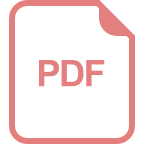











