x = torch.cat([x0, x1, x2, x3], -1) x = x.view(B, -1, 4 * C)
时间: 2023-10-10 08:05:57 浏览: 148
这段代码的作用是将四个张量 `x0`, `x1`, `x2`, `x3` 沿着最后一个维度拼接起来,形成一个新的张量 `x`。然后将 `x` 进行形状变换,将第二个维度展开为 `-1`(自动计算),第三个维度变为 `4*C`。最终得到的张量 `x` 的形状为 `(B, N, 4*C)`,其中 `B` 表示 batch size,`N` 表示第二个维度展开后的长度,`C` 表示 `x0`, `x1`, `x2`, `x3` 张量的最后一个维度的长度。
相关问题
x = torch.cat([x0, x1, x2, x3], -1) # B H/2 W/2 4*C 分析代码 给出案例
这段代码使用 PyTorch 中的 `torch.cat()` 函数将四个张量 `x0, x1, x2, x3` 沿着最后一个维度(即 `-1`)进行拼接,生成一个新的张量 `x`。具体来说,假设 `x0, x1, x2, x3` 的形状为 `B H/2 W/2 C`,则拼接后的张量 `x` 的形状为 `B H/2 W/2 4*C`。其中,`B` 表示 batch size,`H` 和 `W` 分别表示图像的高度和宽度,`C` 表示通道数。
这段代码通常出现在神经网络中的某个卷积层的实现中。在卷积层中,通常会将输入张量沿通道维度进行分组,每个组内的通道数相同,而不同组之间的通道数可以不同。在这种情况下,可以将每个组的输入张量分别经过一些卷积操作后,再使用 `torch.cat()` 函数将它们拼接起来,作为整个卷积层的输出。这个例子中,四个张量的通道数相同,因此可以将它们直接拼接。
例如,假设 `x0, x1, x2, x3` 分别表示一个大小为 `B H/2 W/2 C` 的图像经过不同的卷积操作后得到的四个特征图,现在需要将这四个特征图拼接成一个大小为 `B H/2 W/2 4*C` 的特征张量,可以使用上述代码实现:
```python
import torch
# 假设 x0, x1, x2, x3 分别表示四个大小为 B H/2 W/2 C 的特征图
x0 = torch.randn((2, 16, 16, 32))
x1 = torch.randn((2, 16, 16, 32))
x2 = torch.randn((2, 16, 16, 32))
x3 = torch.randn((2, 16, 16, 32))
# 使用 torch.cat() 函数将四个特征图拼接起来
x = torch.cat([x0, x1, x2, x3], -1)
# 输出拼接后的特征张量的大小
print(x.shape) # 输出: torch.Size([2, 16, 16, 128])
```
Traceback (most recent call last): File "E:/pycharm/AHEcode/train.py", line 229, in <module> outputs = model(images) File "E:\conda\CONDA\envs\hu-torch\lib\site-packages\torch\nn\modules\module.py", line 1130, in _call_impl return forward_call(*input, **kwargs) File "E:/pycharm/AHEcode/train.py", line 63, in forward x_final = torch.cat([x3_flat, lbp_output], dim=1) # 将 x3_flat 和 lbp_output 拼接 RuntimeError: Sizes of tensors must match except in dimension 1. Expected size 6272 but got size 8 for tensor number 1 in the list.完整的代码如下: def forward(self, x): x1 = F.relu(self.bn1(torch.cat([self.conv1_3x3(x), self.conv1_5x5(x), self.conv1_7x7(x)], dim=1))) x1 = F.max_pool2d(x1, 2) x2 = F.relu(self.bn2(torch.cat([self.conv2_3x3(x1), self.conv2_5x5(x1), self.conv2_7x7(x1)], dim=1))) x2 = F.max_pool2d(x2, 2) x3 = F.relu(self.bn3(torch.cat([self.conv3_3x3(x2), self.conv3_5x5(x2), self.conv3_7x7(x2)], dim=1))) x3 = F.max_pool2d(x3, 2) x3_flat = x3.view(-1, 768) print(f'x3_flat size: {x3_flat.size()}') clahe_output = self.clahe_module(x) print(clahe_output.shape) lbp_output = self.lbp_layer(clahe_output) print(f'lbp_output size: {lbp_output.size()}') lbp_output = lbp_output.to(x3_flat.device) # print(f'lbp_output expanded size: {lbp_output.size()}') x_final = torch.cat([x3_flat, lbp_output], dim=1) # 将 x3_flat 和 lbp_output 拼接 output = self.fc(x_final) # 全连接层得到最终的输出 return output
这个错误是因为在 torch.cat 操作中,除了第 1 维度以外,其他维度的大小应该是相同的,但是在你的代码中,x3_flat 的大小是 [batch_size, 768],而 lbp_output 的大小是 [batch_size, 1, height, width],没有经过展平操作,因此维度不匹配。你可以将 lbp_output 展平成 [batch_size, height*width],然后再进行拼接操作。修改后的代码如下:
```
def forward(self, x):
x1 = F.relu(self.bn1(torch.cat([self.conv1_3x3(x), self.conv1_5x5(x), self.conv1_7x7(x)], dim=1)))
x1 = F.max_pool2d(x1, 2)
x2 = F.relu(self.bn2(torch.cat([self.conv2_3x3(x1), self.conv2_5x5(x1), self.conv2_7x7(x1)], dim=1)))
x2 = F.max_pool2d(x2, 2)
x3 = F.relu(self.bn3(torch.cat([self.conv3_3x3(x2), self.conv3_5x5(x2), self.conv3_7x7(x2)], dim=1)))
x3 = F.max_pool2d(x3, 2)
x3_flat = x3.view(-1, 768)
print(f'x3_flat size: {x3_flat.size()}')
clahe_output = self.clahe_module(x)
print(clahe_output.shape)
lbp_output = self.lbp_layer(clahe_output)
lbp_output = lbp_output.view(lbp_output.size(0), -1) # 将 lbp_output 展平
print(f'lbp_output size: {lbp_output.size()}')
lbp_output = lbp_output.to(x3_flat.device)
x_final = torch.cat([x3_flat, lbp_output], dim=1) # 将 x3_flat 和 lbp_output 拼接
output = self.fc(x_final) # 全连接层得到最终的输出
return output
```
这样修改后,应该就可以正常运行了。
阅读全文
相关推荐
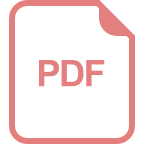
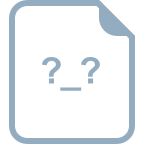
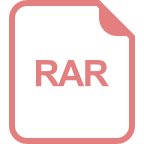
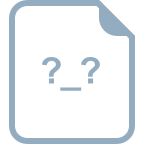
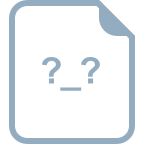
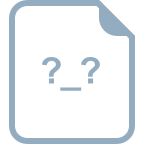
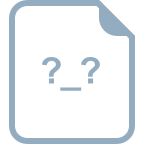
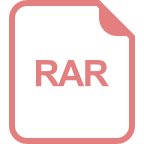
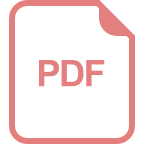
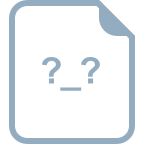
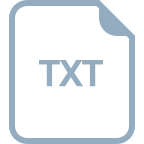
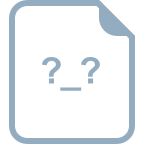
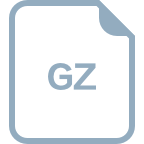