在vue中,我想实现学生端上交作业,可以上传附件,如docx、pdf等类型,同时教师端可以查看学生作业,并预览学生上传的附件,请告诉我使用el-upload组件的学生端和教师端代码,还有告诉我后端springboot对于附件的存储代码和方式
时间: 2024-04-22 15:22:29 浏览: 129
好的,针对您的问题,我可以给您提供一些思路和示例代码,帮助您实现这个功能。
首先是学生端和教师端的代码,我们可以使用 `el-upload` 组件来实现文件上传功能。在学生端,我们可以这样写:
```html
<template>
<el-upload
class="upload-demo"
action="/upload"
:on-success="handleSuccess"
:before-upload="beforeUpload"
:file-list="fileList"
:limit="1"
:auto-upload="false"
>
<el-button slot="trigger" size="small" type="primary">选择文件</el-button>
<el-button
style="margin-left: 10px;"
size="small"
type="success"
@click="uploadFile"
:disabled="fileList.length === 0"
>
上传文件
</el-button>
</el-upload>
</template>
<script>
export default {
data() {
return {
fileList: [],
};
},
methods: {
beforeUpload(file) {
const isPDF = file.type === 'application/pdf';
const isDOCX = file.type === 'application/vnd.openxmlformats-officedocument.wordprocessingml.document';
const isLt5M = file.size / 1024 / 1024 < 5;
if (!isPDF && !isDOCX) {
this.$message.error('只能上传 PDF 或 DOCX 格式的文件');
return false;
}
if (!isLt5M) {
this.$message.error('上传文件大小不能超过 5MB');
return false;
}
this.fileList = [file];
return false;
},
handleSuccess(response) {
this.$message.success('上传成功');
this.fileList = [];
},
uploadFile() {
this.$refs.upload.submit();
},
},
};
</script>
```
在教师端,我们可以使用类似的代码来展示已上传的文件,并提供下载链接:
```html
<template>
<div>
<el-upload
class="upload-demo"
action="/download"
:file-list="fileList"
:limit="1"
:auto-upload="false"
>
<el-button slot="trigger" size="small">查看文件</el-button>
</el-upload>
<el-button
style="margin-left: 10px;"
size="small"
type="success"
@click="downloadFile"
:disabled="fileList.length === 0"
>
下载文件
</el-button>
</div>
</template>
<script>
export default {
data() {
return {
fileList: [],
downloadUrl: '',
};
},
mounted() {
// 模拟已上传的文件
this.fileList = [
{
name: '作业.docx',
url: 'https://www.example.com/homework.docx',
},
];
},
methods: {
downloadFile() {
window.open(this.fileList[0].url);
},
},
};
</script>
```
接下来是后端 Spring Boot 的代码,我们可以使用 `MultipartFile` 类型来接收上传的文件,并使用 `java.nio.file.Files` 类将文件保存到本地:
```java
@PostMapping("/upload")
public ResponseEntity<String> uploadFile(@RequestParam("file") MultipartFile file) {
// 获取文件名
String fileName = file.getOriginalFilename();
// 获取文件存储路径
String filePath = "/path/to/files/" + fileName;
// 将文件保存到本地
try {
Files.write(Paths.get(filePath), file.getBytes());
} catch (IOException e) {
e.printStackTrace();
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body("文件上传失败");
}
return ResponseEntity.ok("文件上传成功");
}
@GetMapping("/download")
public ResponseEntity<Resource> downloadFile() {
// 获取文件存储路径
String filePath = "/path/to/files/作业.docx";
// 将文件读取为 Resource
Resource resource;
try {
resource = new UrlResource(Paths.get(filePath).toUri());
} catch (MalformedURLException e) {
e.printStackTrace();
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).build();
}
return ResponseEntity.ok()
.header(HttpHeaders.CONTENT_DISPOSITION, "attachment; filename=\"" + resource.getFilename() + "\"")
.body(resource);
}
```
在这个例子中,我们将文件保存到了 `/path/to/files/` 目录下,并提供了一个 `/download` 接口来下载文件。需要注意的是,在生产环境中,我们应该将文件保存到更安全的位置,并实现更严格的访问控制。
希望这些代码能对您有所帮助。如果您还有其他问题,欢迎继续提问。
阅读全文
相关推荐
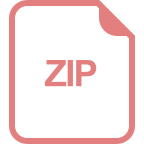
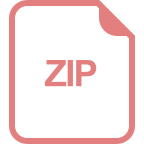

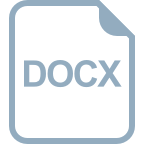
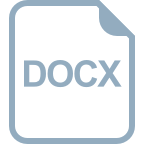
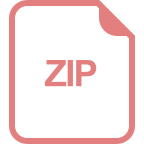
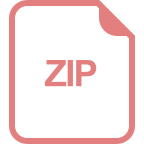
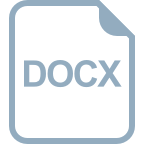
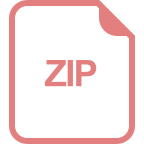
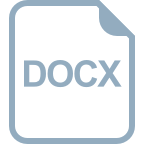
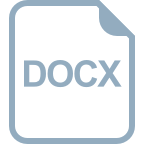
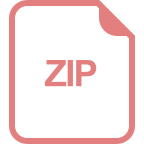
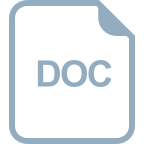