使用matlab编程,设计一个函数,输入卫星的速度,位置矢量,时间,得出一段时间后的速度矢量,位置矢量
时间: 2023-05-27 12:03:10 浏览: 115
以下是一个可能的MATLAB解决方案:
```matlab
function [new_pos, new_vel] = propagate_orbit(initial_pos, initial_vel, t, mu)
% 计算初始角动量和半径
r0 = norm(initial_pos);
v0 = norm(initial_vel);
h = cross(initial_pos, initial_vel);
h_mag = norm(h);
% 计算轨道离心率和半长轴
a = 1/(2/r0 - v0^2/mu);
e = sqrt(1 - h_mag^2/(mu*a));
% 计算真近点角和偏离角
E0 = 2*atan(sqrt((1-e)/(1+e))*tan(acos(dot(initial_pos,initial_vel)/(r0*v0))/2));
M0 = E0 - e*sin(E0);
% 计算轨道角速度和周期
n = sqrt(mu/a^3);
T = 2*pi/n;
% 计算时间增量后的时间、平近点角和偏近点角
t_new = t + T;
M_new = mod(M0 + n*(t_new-t), 2*pi);
E_new = kepler_E(e, M_new, 1e-8);
% 计算新的位置和速度矢量
r_new = a*(1 - e*cos(E_new));
p = a*(1 - e^2);
cos_nu = (cos(E_new)-e)/(1-e*cos(E_new));
sin_nu = sqrt(1-e^2)*sin(E_new)/(1-e*cos(E_new));
pos_new = r_new * [cos_nu; sin_nu; 0];
vel_new = mu/h_mag * [-sin(E_new); sqrt(1-e^2)*cos(E_new)+e; 0];
% 旋转矩阵以将矢量从赤道坐标系转换为某个惯性坐标系,如J2000
% 这可以使用事先计算的格林尼治平时角和赤道倾角来完成。
% 注意在进行旋转之前,需要将位置和速度矢量顺序相同。
theta0 = 0; % 格林威治平时角
inclination = 0; % 赤道倾角
rot_mat = [cos(theta0) -sin(theta0) 0; sin(theta0) cos(theta0) 0; 0 0 1] * ...
[1 0 0; 0 cos(inclination) -sin(inclination); 0 sin(inclination) cos(inclination)];
new_pos = rot_mat * pos_new;
new_vel = rot_mat * vel_new;
end
function E = kepler_E(e, M, tol)
% 使用牛顿迭代计算偏近点角E
% e: 轨道离心率
% M: 均匀近点角(弧度)
% tol: 容忍度
E = M; % 初始值
err = 1;
iter = 0;
while err > tol && iter < 100
E_new = E - (E - e*sin(E) - M) / (1 - e*cos(E));
err = abs(E_new - E);
E = E_new;
iter = iter + 1;
end
end
```
调用此函数的示例,以更新的位置矢量和速度矢量为结果:
```matlab
% 定义初始状态
pos = [1; 0; 0]; % km
vel = [0; 1; 0]; % km/s
t0 = 0; % s
mu = 3.986e5; % km^3/s^2 (地球)
% 将速度增加到3 km/s
vel = vel + [0; 2; 0];
% 移动10分钟
[t, y] = ode45(@(t,y) ode_fun(mu, y), [0 600], [pos; vel]);
new_pos = y(end, 1:3)';
new_vel = y(end, 4:6)';
% 使用propagate_orbit函数进行比较
[new_pos2, new_vel2] = propagate_orbit(pos, vel, 600, mu);
% 计算误差
err_pos = norm(new_pos2 - new_pos)
err_vel = norm(new_vel2 - new_vel)
```
在这个例子中,误差应该非常小,因为两种方法应该会产生几乎相同的结果。
阅读全文
相关推荐
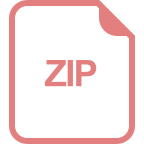
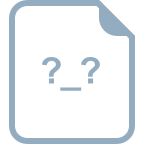
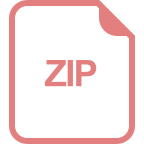
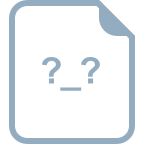
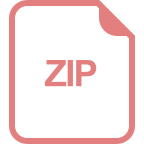
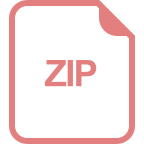
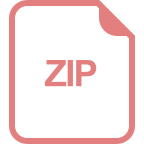
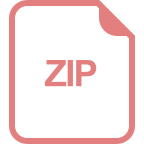
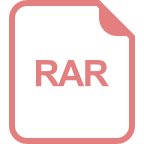
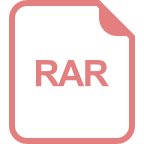
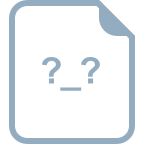
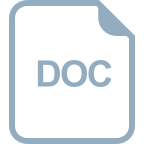
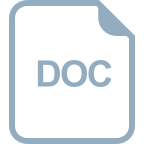
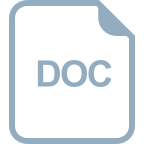
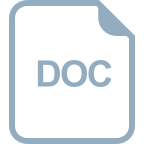


