c++定义一个 Shape 类记录任意形状的位置,并定义一个计算面积的函数和显示图形的形 状及中心位置的函数,这两个函数都是纯虛函数。在 Shape 类的基础上派生出 - ^ Rectangle 类和一个 circle 类,这两个类都有计算面积和是示形状及中心位置的函数。然后在主函数中 用抽象类的指针调用函数来输出相应图形的面积及其显示形状及中心位置。
时间: 2023-12-14 09:39:57 浏览: 141
以下是 C++ 代码实现:
```cpp
#include<iostream>
using namespace std;
// 基类 Shape
class Shape {
protected:
int x, y; // 记录位置
public:
Shape(int x, int y): x(x), y(y) {} // 构造函数
virtual float getArea() = 0; // 纯虚函数,用于计算面积
virtual void display() = 0; // 纯虚函数,用于显示形状及中心位置
};
// 派生类 Rectangle
class Rectangle : public Shape {
private:
int width, height; // 长和宽
public:
Rectangle(int x, int y, int width, int height) : Shape(x, y), width(width), height(height) {} // 构造函数
float getArea() { return width * height; } // 计算面积
void display() { cout << "Rectangle, center: (" << x << "," << y << "), width: " << width << ", height: " << height << endl; } // 显示形状及中心位置
};
// 派生类 Circle
class Circle : public Shape {
private:
int radius; // 半径
public:
Circle(int x, int y, int radius) : Shape(x, y), radius(radius) {} // 构造函数
float getArea() { return 3.14 * radius * radius; } // 计算面积
void display() { cout << "Circle, center: (" << x << "," << y << "), radius: " << radius << endl; } // 显示形状及中心位置
};
int main() {
// 定义抽象类指针
Shape* shape1;
Shape* shape2;
// 创建 Rectangle 对象
Rectangle rectangle(0, 0, 4, 3);
shape1 = &rectangle;
cout << "Rectangle area: " << shape1->getArea() << endl;
shape1->display();
// 创建 Circle 对象
Circle circle(0, 0, 5);
shape2 = &circle;
cout << "Circle area: " << shape2->getArea() << endl;
shape2->display();
return 0;
}
```
输出结果:
```
Rectangle area: 12
Rectangle, center: (0,0), width: 4, height: 3
Circle area: 78.5
Circle, center: (0,0), radius: 5
```
阅读全文
相关推荐
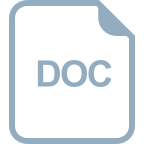
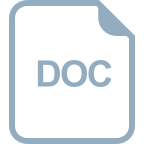
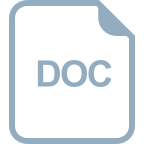

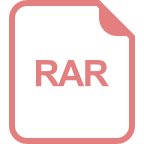
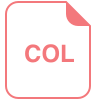
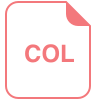
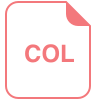
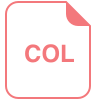
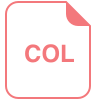
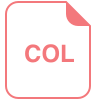


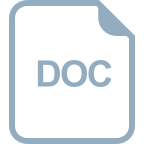
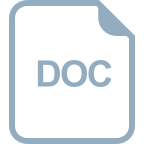