让文本随着时间滚动
时间: 2023-08-02 21:10:37 浏览: 78
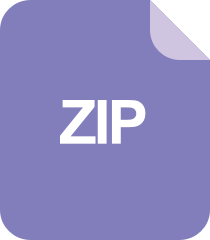
文字滚动
如果你想让文本随着时间滚动,可以使用Android系统提供的`TextSwitcher`控件和`Handler`类,实现一个简单的上下滚动效果。
1. 首先,在布局文件中使用`TextSwitcher`控件,将它放在一个`FrameLayout`中。`TextSwitcher`控件可以用来切换文本内容,这里我们将它用来显示滚动的文本。
示例代码如下:
```xml
<FrameLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextSwitcher
android:id="@+id/text_switcher"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
</FrameLayout>
```
2. 接下来,在代码中,你可以使用`Handler`类来定时更新`TextSwitcher`的文本内容,从而实现文本的滚动效果。
示例代码如下:
```java
TextSwitcher textSwitcher = findViewById(R.id.text_switcher);
textSwitcher.setFactory(new ViewSwitcher.ViewFactory() {
@Override
public View makeView() {
TextView textView = new TextView(MainActivity.this);
textView.setTextSize(20);
textView.setTextColor(Color.BLACK);
textView.setGravity(Gravity.CENTER);
return textView;
}
});
String[] textArray = new String[]{"这是第一行文本", "这是第二行文本", "这是第三行文本"};
int currentIndex = 0;
Handler handler = new Handler();
Runnable updateTextRunnable = new Runnable() {
@Override
public void run() {
textSwitcher.setText(textArray[currentIndex]);
currentIndex = (currentIndex + 1) % textArray.length;
handler.postDelayed(this, 2000);
}
};
handler.post(updateTextRunnable);
```
这段代码中,我们首先使用`TextSwitcher`的`setFactory()`方法设置了一个`ViewFactory`,用来创建`TextSwitcher`的子视图。在这个示例中,我们创建了一个`TextView`,并设置了一些样式属性。你可以根据自己的需求来设置。
接着,我们定义了一个`String`数组来存储要滚动的文本内容。然后,我们使用`Handler`类和`Runnable`接口来实现定时更新文本内容的功能。在这个示例中,我们每隔2秒钟更新一次文本内容,滚动到下一行。注意,我们使用了`handler.postDelayed()`方法来延迟2秒钟再次更新文本内容。
通过这种方式,你就可以实现一个简单的文本滚动效果了。如果你想控制滚动的速度或者方向,可以调整`Runnable`中的`postDelayed()`方法的参数。
阅读全文
相关推荐
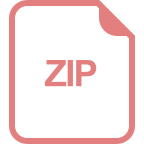
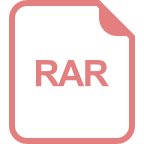
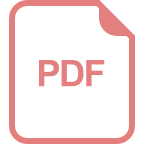
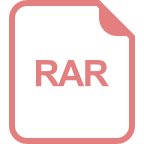
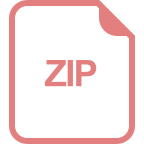
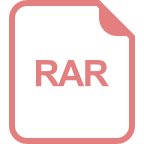
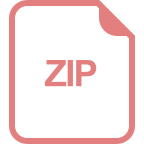
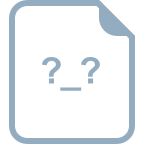
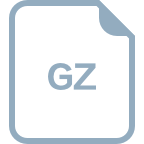
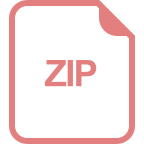
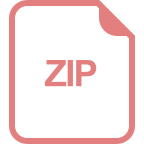
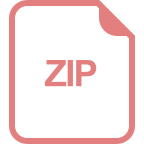
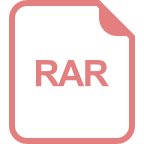
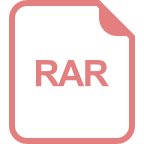
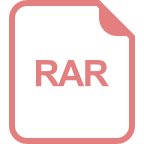
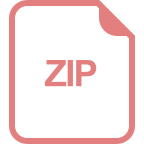