Use an int template nontype parameter numberOfElements and a type parameter elementType to help create a template for the Array class. This template will enable Array objects to be instantiated with a specified number of elements of a specified element type at compile time.
时间: 2024-03-07 15:49:48 浏览: 50
Sure! Here is an example implementation of the Array class template:
```
template <typename T, int N>
class Array {
private:
T data[N];
public:
T& operator[](int index) {
return data[index];
}
};
```
In this implementation, the class template takes two parameters: `T` for the element type and `N` for the number of elements. The `data` member variable is an array of `N` elements of type `T`.
The `operator[]` function allows you to access elements of the array using the index operator. For example:
```
Array<int, 5> myArray;
for (int i = 0; i < 5; i++) {
myArray[i] = i;
}
for (int i = 0; i < 5; i++) {
std::cout << myArray[i] << " ";
}
```
This will create an `Array` object with 5 `int` elements, initialize them with the values 0 to 4, and then print them out.
阅读全文
相关推荐
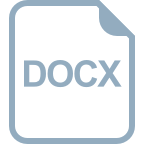
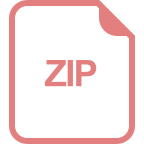
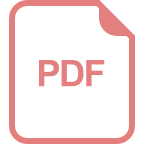
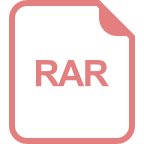

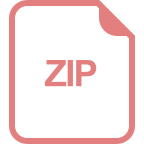
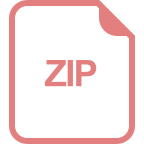
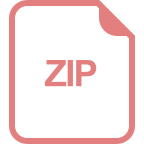
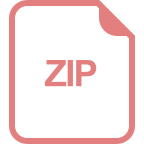
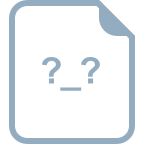
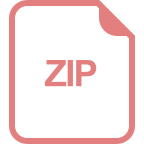