如何使用qt读取dwg文件中坐标信息
时间: 2023-08-02 11:12:16 浏览: 665
要在Qt中读取DWG文件中的坐标信息,可以使用DWG阅读器库,例如ODA的Teigha库。以下是一些示例代码,演示如何使用Teigha库和Qt读取DWG文件中的坐标信息:
```cpp
#include <QtWidgets/QApplication>
#include <QtWidgets/QMainWindow>
#include <OdaCommon.h>
#include <Gi/GiViewport.h>
#include <Gi/GiGeometrySimplifier.h>
#include <Gi/GiSubEntityTraits.h>
#include <Gs/Gs.h>
#include <Ge/GeMatrix3d.h>
#include <RxVariantValue.h>
#include <RxObjectImpl.h>
#include <RxModule.h>
class DwgViewer : public QMainWindow, public OdGiGeometrySimplifier
{
public:
DwgViewer(QWidget *parent = 0)
: QMainWindow(parent)
{
OdRxModulePtr pMod = ::odrxDynamicLinker()->loadModule(OdString("TD_Root"));
if (pMod.isNull())
throw std::runtime_error("Cannot load Teigha module");
OdRxModulePtr pRenderMod = ::odrxDynamicLinker()->loadModule(OdString("TD_Gs"));
if (pRenderMod.isNull())
throw std::runtime_error("Cannot load Teigha render module");
m_pGsDevice = OdGsDevicePtr(OdGsDevice::createObject(), OdRxObjectImpl<OdGsDevice>);
m_pGsView = OdGsViewPtr(OdGsView::createObject(), OdRxObjectImpl<OdGsView>);
m_pGsView->setViewport(m_pGsDevice->createViewport());
this->setCentralWidget(m_pGsView->viewport()->widget());
this->resize(800, 600);
OdDbDatabasePtr pDb = OdDbDatabase::createObject();
if (pDb.isNull())
throw std::runtime_error("Cannot create database");
if (pDb->readDwgFile(OdString("<filepath>")) != eOk)
throw std::runtime_error("Cannot read DWG file");
OdDbBlockTablePtr pBlockTable = pDb->getBlockTable();
if (!pBlockTable.isNull())
{
OdDbBlockTableRecordPtr pRecord;
if (pBlockTable->getAt(OdString("Model_Space"), pRecord) != eOk)
throw std::runtime_error("Cannot get Model_Space");
OdDbBlockTableRecordIteratorPtr pIterator = pRecord->newIterator();
while (!pIterator->done())
{
OdDbEntityPtr pEntity = pIterator->entity();
if (!pEntity.isNull())
{
pEntity->subOpen();
pEntity->subWorldDraw(this);
pEntity->subClose();
}
pIterator->step();
}
}
m_pGsView->setViewportBorderWidth(0);
m_pGsView->add(OdGsDevicePtr(m_pGsDevice));
m_pGsDevice->setBackgroundColor(ODRGB(255, 255, 255));
m_pGsView->invalidate();
m_pGsView->update();
}
virtual void polylineOut(OdInt32 nbPoints, const OdGePoint3d* pVertexList)
{
// 处理每个多边形的坐标信息
for (int i = 0; i < nbPoints; ++i)
{
OdGePoint3d point = pVertexList[i];
// 处理坐标点,例如打印它们
qDebug() << point.x << point.y << point.z;
}
}
private:
OdGsDevicePtr m_pGsDevice;
OdGsViewPtr m_pGsView;
};
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
try
{
DwgViewer viewer;
viewer.show();
a.exec();
}
catch (const std::exception& ex)
{
qDebug() << ex.what();
return 1;
}
return 0;
}
```
请注意,上面的示例代码仅演示了如何读取DWG文件中的坐标信息,并打印出来。您可以根据您的实际需求,对代码进行更改和扩展。
阅读全文
相关推荐
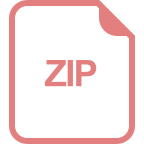

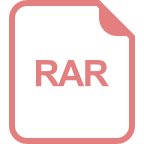
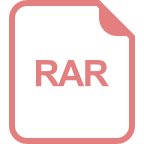
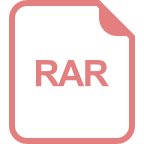
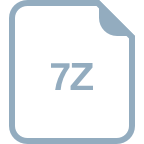
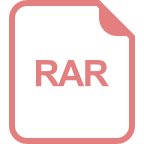

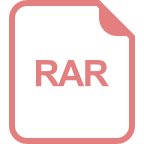
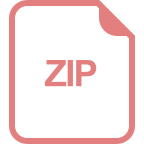