2.定义一个Student类,要求如下: 属性:id、name; 方法:构造方法,set和get方法,其中setid(String id)若id长度大于10,则抛出LengthException,若id中含有字母字符,则抛出LetterException 。 编写自定义LengthException和LetterException异常类。 编写Student类。在setid方法体中抛出LengthException或LetterException异常类 编写类ExceptionTest3: 3. student对象的信息存储到E://object.txt中。
时间: 2024-03-06 16:48:41 浏览: 28
好的,这是一个关于Java编程的问题。
首先我们需要定义一个Student类,并且在其中包含id和name两个属性,并且要求我们实现get和set方法,并在setid方法中抛出LengthException和LetterException异常。
以下是Student类的代码实现:
```java
public class Student {
private String id;
private String name;
public Student(String id, String name) {
this.id = id;
this.name = name;
}
public String getId() {
return id;
}
public void setId(String id) throws LetterException, LengthException {
if (id.length() > 10) {
throw new LengthException("ID长度不能超过10位!");
} else if (id.matches(".*[a-zA-Z]+.*")) {
throw new LetterException("ID中不能含有字母字符!");
}
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
```
在上面的代码中,我们定义了一个Student类,并且在setId方法中进行了判断,如果ID的长度超过了10位,我们就会抛出LengthException异常,如果ID中含有字母字符,就会抛出LetterException异常。我们需要自定义这两个异常类:
```java
public class LengthException extends Exception {
public LengthException(String message) {
super(message);
}
}
public class LetterException extends Exception {
public LetterException(String message) {
super(message);
}
}
```
接下来,我们需要编写一个ExceptionTest3类来测试我们的Student类,并将student对象的信息存储到E://object.txt中。
以下是ExceptionTest3类的代码实现:
```java
import java.io.FileOutputStream;
import java.io.ObjectOutputStream;
public class ExceptionTest3 {
public static void main(String[] args) {
Student student = new Student("1234567890", "张三");
try {
student.setId("1234567890a");
} catch (LengthException e) {
e.printStackTrace();
} catch (LetterException e) {
e.printStackTrace();
}
try {
FileOutputStream fos = new FileOutputStream("E://object.txt");
ObjectOutputStream oos = new ObjectOutputStream(fos);
oos.writeObject(student);
oos.close();
fos.close();
System.out.println("Student对象已经被存储到E://object.txt中。");
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
在上面的代码中,我们创建了一个Student对象,并且在setId方法中传入了一个包含字母字符的ID,这样就会抛出LetterException异常。接下来,我们将这个对象存储到了E://object.txt文件中。
这样就完成了这个问题的解答。
相关推荐
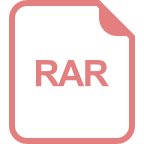
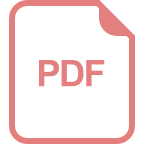
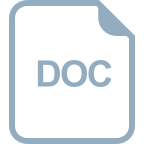














