使用c语言写一个景点录入,删除的函数
时间: 2024-02-27 18:56:45 浏览: 26
好的,以下是一个简单的景点录入、删除程序的示例代码,供参考:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_SIZE 100
struct Spot {
char name[50];
char city[20];
int rating;
};
struct Spot spots[MAX_SIZE];
int num_spots = 0;
void addSpot() {
if (num_spots >= MAX_SIZE) {
printf("无法添加,已达到最大数量!\n");
return;
}
printf("请输入景点名称:");
scanf("%s", spots[num_spots].name);
printf("请输入所在城市:");
scanf("%s", spots[num_spots].city);
printf("请输入评分(1-10):");
scanf("%d", &spots[num_spots].rating);
num_spots++;
printf("已添加!\n");
}
void deleteSpot() {
if (num_spots <= 0) {
printf("无法删除,没有景点!\n");
return;
}
char name[50];
printf("请输入要删除的景点名称:");
scanf("%s", name);
int index = -1;
for (int i = 0; i < num_spots; i++) {
if (strcmp(spots[i].name, name) == 0) {
index = i;
break;
}
}
if (index == -1) {
printf("无法删除,未找到该景点!\n");
return;
}
for (int i = index; i < num_spots - 1; i++) {
spots[i] = spots[i + 1];
}
num_spots--;
printf("已删除!\n");
}
int main() {
int choice = 0;
while (1) {
printf("请选择操作:\n");
printf("1. 添加景点\n");
printf("2. 删除景点\n");
printf("3. 退出\n");
printf("请选择:");
scanf("%d", &choice);
switch (choice) {
case 1:
addSpot();
break;
case 2:
deleteSpot();
break;
case 3:
printf("谢谢使用!\n");
return 0;
default:
printf("无效的选择!\n");
}
printf("\n");
}
}
```
这个程序使用了一个结构体 `Spot` 来存储每个景点的名称、所在城市、评分。`MAX_SIZE` 定义了最大景点数量,可以根据需要进行修改。
程序中包含两个主要函数 - `addSpot()` 和 `deleteSpot()`。`addSpot()` 用于添加新的景点,会提示用户输入名称、城市和评分,然后将其添加到 `spots` 数组中。`deleteSpot()` 用于删除指定景点,会提示用户输入要删除的景点名称,然后在 `spots` 数组中查找该景点并删除。
主函数中使用一个循环来不断接收用户的选择,直到用户选择退出程序。在每次用户选择操作后,都会打印一个空行,以便提高程序输出的可读性。
当然,这只是一个简单的示例程序,如果你需要实现更多功能,可以根据需要进行修改和扩展。
相关推荐
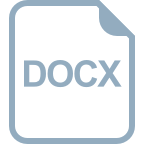












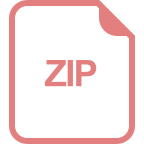
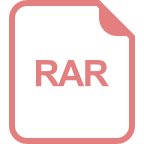