Spring Security OAuth2 整合
时间: 2024-02-22 20:49:25 浏览: 119
OAuth2 是什么?它有什么作用?
OAuth2 是一个开放标准协议,用于授权访问第三方应用程序。它允许用户授权第三方应用程序访问他们存储在另一个服务提供者上的受保护资源,而不需要将其凭证提供给第三方应用程序。使用 OAuth2,用户可以授权第三方应用程序仅使用他们授权的资源,而不需要共享他们的凭证。这可以增强用户的安全性和隐私。而 Spring Security OAuth2 则是基于 Spring Security 的一个 OAuth2 解决方案,它可以帮助我们轻松实现 OAuth2 的授权和认证功能。
相关问题
spring security oauth2整合zuul网关,通过网关获取授权码 访问xxx/oauth/authorize时,未登录直接跳到oauth2认证服务的login地址上去,点击登录就报404
Spring Security OAuth2整合Zuul网关时,通常涉及到身份验证流程的拦截和转发。当用户访问`/xxx/oauth/authorize`路径并未经身份验证时,Zuul会根据配置自动将请求转发到OAuth服务器的登录页面。如果遇到404错误,这可能是以下几个原因:
1. **URL配置问题**:确保Zuul的路由配置指向了正确的OAuth认证服务的`/login`地址。检查`zuul.routes.oauth2.path`是否设置为`/login`。
```yaml
zuul:
routes:
oauth2:
path: /oauth/**
serviceId: oauth-service
```
2. **服务提供者配置**:确认你的OAuth服务端配置中,登录路径(`redirect_uri`)是否正确配置了返回地址,即`http://your-gateway-url/login`。
3. **跨域问题**:如果OAuth服务器不在同一个域名下,需要在`OAuth2ClientContextFilter`中处理CORS请求头,允许来自Zuul的跨域访问。
4. **Zuul全局过滤器顺序**:检查`zuul.filters.order`中是否有处理身份验证的过滤器,如`spring-security-oauth2-autoconfigure`下的过滤器。如果没有,可能需要调整它们的位置。
5. **Spring Security配置**:确保你的Spring Security配置中正确地设置了`SecurityFilterChain`和`AuthenticationEntryPoint`,以便在无权限时能正常重定向。
如果你已经检查过上述点,仍然存在问题,可以尝试增加一些日志信息来定位问题,比如查看Zuul、OAuth服务以及Spring Security的日志输出。同时,
springboot整合spring security oauth2 代码示例
以下是一个简单的 Spring Boot 整合 Spring Security OAuth2 的代码示例:
1. 添加依赖
在 pom.xml 文件中添加以下依赖:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.security.oauth</groupId>
<artifactId>spring-security-oauth2</artifactId>
<version>2.3.3.RELEASE</version>
</dependency>
```
2. 配置 OAuth2 客户端
在 application.yml 文件中添加以下配置:
```
security:
oauth2:
client:
clientId: your-client-id
clientSecret: your-client-secret
accessTokenUri: https://your-auth-server.com/oauth/token
userAuthorizationUri: https://your-auth-server.com/oauth/authorize
clientAuthenticationScheme: form
scope: read write
```
3. 配置 Spring Security
创建一个继承自 WebSecurityConfigurerAdapter 的类,并添加以下配置:
```
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/oauth/**").permitAll()
.anyRequest().authenticated()
.and()
.formLogin().permitAll()
.and()
.csrf().disable();
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("user").password("{noop}password").roles("USER");
}
}
```
4. 创建资源服务器
创建一个继承自 ResourceServerConfigurerAdapter 的类,并添加以下配置:
```
@Configuration
@EnableResourceServer
public class ResourceServerConfig extends ResourceServerConfigurerAdapter {
@Override
public void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/api/**").authenticated()
.anyRequest().permitAll();
}
@Override
public void configure(ResourceServerSecurityConfigurer resources) throws Exception {
resources.resourceId("resource-id");
}
}
```
5. 创建控制器
创建一个简单的控制器,用于测试 OAuth2 认证:
```
@RestController
public class TestController {
@GetMapping("/api/test")
public String test() {
return "Hello, World!";
}
}
```
6. 运行应用程序
现在你可以运行应用程序,并访问 http://localhost:8080/api/test 进行测试。如果你没有提供正确的 OAuth2 认证信息,你将会收到一个 401 Unauthorized 错误。
阅读全文
相关推荐
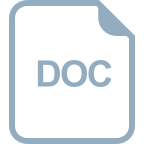
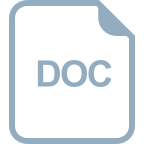
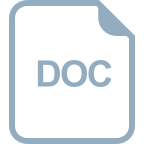
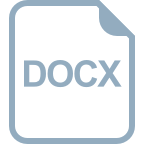
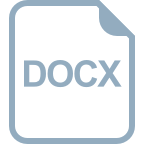
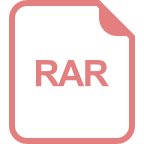
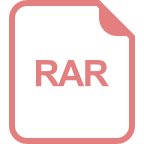
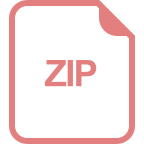
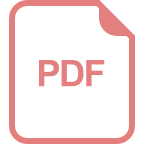
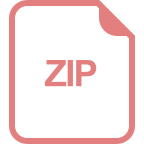
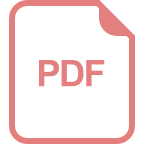
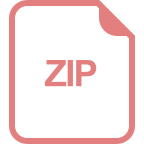
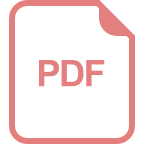
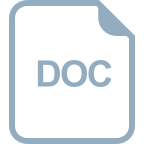
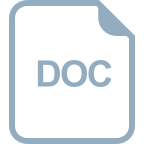
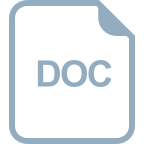
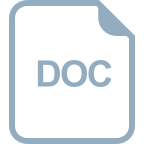