JAVA中将WORD转换为HTML导入到UEDITOR编辑器中
时间: 2024-03-02 08:51:29 浏览: 186
在Java中将Word转换为HTML需要使用Apache POI和JodConverter库。而将HTML导入到UEditor编辑器中,需要在前端使用UEditor富文本编辑器,并在后端使用Java Web框架(如Spring MVC)来处理上传文件和保存HTML内容。
以下是一个简单的Java代码示例,演示如何将Word文档转换为HTML,并将HTML保存到服务器上的文件中:
```java
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import org.apache.poi.hwpf.HWPFDocument;
import org.jodconverter.JodConverter;
import org.jodconverter.office.LocalOfficeManager;
import org.jodconverter.office.OfficeException;
import org.jodconverter.office.OfficeManager;
public class WordToHtmlConverter {
public static void main(String[] args) {
// Start the office manager
OfficeManager officeManager = LocalOfficeManager.builder().officeHome("/usr/lib/libreoffice").install().build();
try {
officeManager.start();
// Load the Word document
HWPFDocument document = new HWPFDocument(new FileInputStream("document.doc"));
// Create a temporary file for the HTML output
File tempFile = File.createTempFile("document", ".html");
// Convert the document to HTML
JodConverter.convert(document).to(tempFile).execute();
// Save the HTML content to a file on the server
FileOutputStream fos = new FileOutputStream("output.html");
fos.write(Files.readAllBytes(tempFile.toPath()));
fos.close();
// Delete the temporary file
tempFile.delete();
} catch (IOException | OfficeException e) {
e.printStackTrace();
} finally {
// Stop the office manager
officeManager.stop();
}
}
}
```
在上述代码中,我们使用JodConverter将Word文档转换为HTML,并将HTML内容保存到服务器上的文件中。然后,我们可以在Java Web应用程序中使用Spring MVC等框架来处理上传文件,并将HTML内容保存到数据库中。最后,在前端使用UEditor富文本编辑器时,我们可以将HTML内容加载到编辑器中,让用户进行编辑和保存。
阅读全文
相关推荐
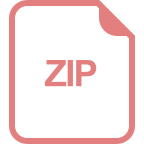
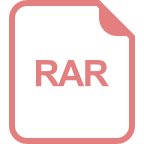
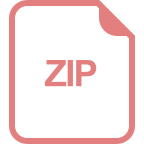
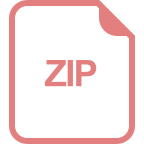
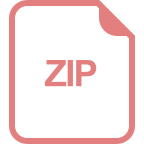
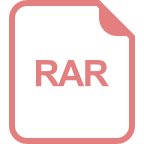
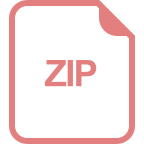
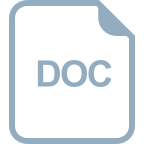
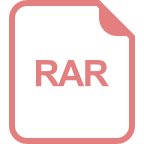
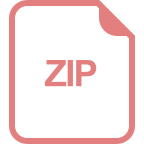