写一段springboot代码
时间: 2023-03-15 11:18:14 浏览: 52
@SpringBootApplication
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}}
相关问题
写一段springboot代码,用于调整jar包加载顺序
好的,以下是一段简单的Spring Boot代码,用于调整Jar包加载顺序:
```
@SpringBootApplication
public class MyApp {
public static void main(String[] args) {
SpringApplication app = new SpringApplication(MyApp.class);
// 获取Classloader
ClassLoader classLoader = MyApp.class.getClassLoader();
// 获取所有Jar包的URL,并根据需要重新排序
List<URL> urls = Collections.list(classLoader.getResources("META-INF/spring.factories"));
Collections.reverse(urls);
// 设置Jar包加载顺序
URL[] sortedUrls = urls.toArray(new URL[0]);
app.setDefaultProperties(Collections.singletonMap("spring.jar.order", StringUtils.arrayToCommaDelimitedString(sortedUrls)));
app.run(args);
}
}
```
在上面的代码中,我们使用了Spring Boot的`SpringApplication`类来启动我们的应用程序。在创建`SpringApplication`实例后,我们通过`getClassLoader()`方法获取到了当前应用程序的ClassLoader。然后,我们通过调用ClassLoader的`getResources()`方法,获取所有的Jar包URL,并对它们进行了排序。最后,我们使用`setDefaultProperties()`方法将排序后的Jar包URL设置为应用程序的默认属性。这样,Spring Boot就会按照我们指定的顺序加载Jar包。
写一段springboot2代码
好的,以下是一个简单的 Spring Boot 2 应用程序的示例代码:
```java
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
@RestController
public class MyApp {
public static void main(String[] args) {
SpringApplication.run(MyApp.class, args);
}
@GetMapping("/")
public String hello() {
return "Hello, World!";
}
}
```
这是一个最简单的 Spring Boot 应用程序,它定义了一个 RESTful 的 HTTP GET 请求,将会返回字符串 "Hello, World!"。其中,`@SpringBootApplication` 注解表示这是一个 Spring Boot 应用程序,`@RestController` 注解表示这个类将处理 RESTful 请求,`@GetMapping` 注解定义了一个处理 HTTP GET 请求的方法,使用 "/" 路径映射到该方法。
相关推荐
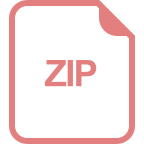
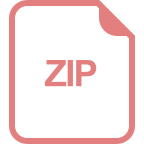
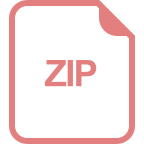












