pyqt5轮播图片一开始就有图
时间: 2024-02-05 09:04:22 浏览: 15
可以使用QTimer和QLabel实现轮播图片功能。首先,将需要显示的图片文件名存储在一个列表中,然后使用QTimer定时器来控制图片的切换。每次定时器时间到达时,更新QLabel的显示图片。
以下是一个简单的示例代码,可以实现从程序开始就有图片显示,以及每隔3秒切换一次图片:
```python
from PyQt5.QtCore import QTimer
from PyQt5.QtGui import QPixmap
from PyQt5.QtWidgets import QApplication, QLabel, QWidget, QVBoxLayout
import sys
class MainWindow(QWidget):
def __init__(self):
super().__init__()
self.setWindowTitle("Image Slider")
self.setGeometry(100, 100, 640, 480)
# 图片文件名列表
self.image_list = ["image1.jpg", "image2.jpg", "image3.jpg"]
# 创建QLabel控件,用来显示图片
self.image_label = QLabel(self)
self.image_label.setPixmap(QPixmap(self.image_list[0]))
# 创建垂直布局,将QLabel添加到布局中
self.layout = QVBoxLayout(self)
self.layout.addWidget(self.image_label)
# 设置定时器,每隔3秒切换一次图片
self.timer = QTimer(self)
self.timer.timeout.connect(self.update_image)
self.timer.start(3000)
def update_image(self):
"""
更新图片
"""
# 获取当前显示的图片在列表中的索引
current_index = self.image_list.index(self.image_label.pixmap().fileName())
# 计算下一张图片的索引
next_index = (current_index + 1) % len(self.image_list)
# 更新QLabel显示的图片
self.image_label.setPixmap(QPixmap(self.image_list[next_index]))
if __name__ == "__main__":
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
```
在该示例代码中,我们将三张图片文件名分别存储在`self.image_list`列表中,然后使用`QLabel`控件来显示图片。在程序运行时,首先将第一张图片显示在`QLabel`控件中,然后使用`QTimer`定时器每隔3秒钟调用`update_image`方法切换图片。在`update_image`方法中,我们获取当前显示的图片的索引,然后计算下一张图片的索引,最后使用`QPixmap`更新`QLabel`控件中显示的图片。
阅读全文
相关推荐
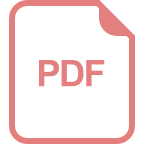
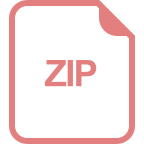
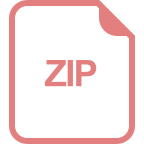
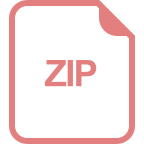
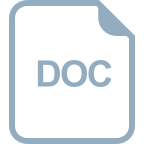
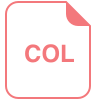

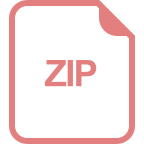
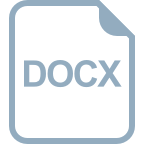
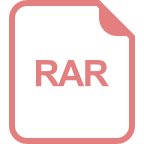
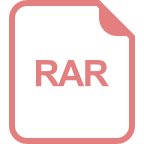
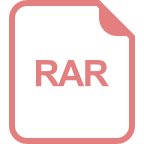
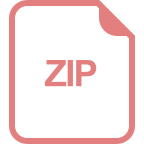
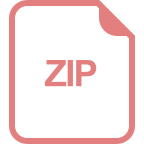
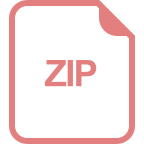