C# 把DataTable A,DataTable B,DataTable C里面相同的序列号的相关的数据用代码提取出来组成一张新表并用dgv显示
时间: 2024-01-21 18:17:38 浏览: 31
假设三个DataTable的结构如下:
DataTable A:
| 序列号 | 名称 | 数量 |
|--------|------|------|
| 1 | A | 10 |
| 2 | B | 20 |
| 3 | C | 30 |
DataTable B:
| 序列号 | 颜色 | 价格 |
|--------|------|------|
| 1 | 红色 | 100 |
| 2 | 黄色 | 200 |
| 4 | 蓝色 | 300 |
DataTable C:
| 序列号 | 重量 | 大小 |
|--------|------|------|
| 1 | 1kg | S |
| 3 | 2kg | M |
| 4 | 3kg | L |
我们需要把三个表中相同序列号的数据提取出来并组成一个新表,可以按照以下步骤进行操作:
1. 创建一个新的DataTable,用于存放提取出来的数据:
```
DataTable newTable = new DataTable();
newTable.Columns.Add("序列号", typeof(int));
newTable.Columns.Add("名称", typeof(string));
newTable.Columns.Add("数量", typeof(int));
newTable.Columns.Add("颜色", typeof(string));
newTable.Columns.Add("价格", typeof(int));
newTable.Columns.Add("重量", typeof(string));
newTable.Columns.Add("大小", typeof(string));
```
2. 遍历DataTable A中的每一行,查找在DataTable B和DataTable C中是否存在相同序列号的行,如果存在,则将三个表中的数据合并到新表中:
```
foreach (DataRow rowA in dataTableA.Rows)
{
int serialNumber = Convert.ToInt32(rowA["序列号"]);
DataRow[] rowsB = dataTableB.Select("序列号 = " + serialNumber);
DataRow[] rowsC = dataTableC.Select("序列号 = " + serialNumber);
if (rowsB.Length > 0 && rowsC.Length > 0)
{
DataRow newRow = newTable.NewRow();
newRow["序列号"] = serialNumber;
newRow["名称"] = rowA["名称"];
newRow["数量"] = rowA["数量"];
newRow["颜色"] = rowsB[0]["颜色"];
newRow["价格"] = rowsB[0]["价格"];
newRow["重量"] = rowsC[0]["重量"];
newRow["大小"] = rowsC[0]["大小"];
newTable.Rows.Add(newRow);
}
}
```
3. 将新表绑定到DataGridView上显示:
```
dataGridView1.DataSource = newTable;
```
完整的代码示例:
```
DataTable newTable = new DataTable();
newTable.Columns.Add("序列号", typeof(int));
newTable.Columns.Add("名称", typeof(string));
newTable.Columns.Add("数量", typeof(int));
newTable.Columns.Add("颜色", typeof(string));
newTable.Columns.Add("价格", typeof(int));
newTable.Columns.Add("重量", typeof(string));
newTable.Columns.Add("大小", typeof(string));
foreach (DataRow rowA in dataTableA.Rows)
{
int serialNumber = Convert.ToInt32(rowA["序列号"]);
DataRow[] rowsB = dataTableB.Select("序列号 = " + serialNumber);
DataRow[] rowsC = dataTableC.Select("序列号 = " + serialNumber);
if (rowsB.Length > 0 && rowsC.Length > 0)
{
DataRow newRow = newTable.NewRow();
newRow["序列号"] = serialNumber;
newRow["名称"] = rowA["名称"];
newRow["数量"] = rowA["数量"];
newRow["颜色"] = rowsB[0]["颜色"];
newRow["价格"] = rowsB[0]["价格"];
newRow["重量"] = rowsC[0]["重量"];
newRow["大小"] = rowsC[0]["大小"];
newTable.Rows.Add(newRow);
}
}
dataGridView1.DataSource = newTable;
```
相关推荐
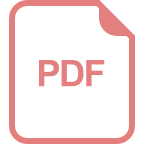














