vue3怎么路由传参并获取传递过来的参数
时间: 2023-05-25 12:03:24 浏览: 789
可以使用Vue Router的props选项实现路由传参,具体步骤如下:
1. 在路由配置中添加props选项,通过设置为true,可以将路由参数作为组件的props传递过去。
```
const router = new VueRouter({
routes: [
{
path: '/user/:id',
name: 'user',
component: User,
props: true
}
]
})
```
2. 在组件中声明props接收路由参数。
```
export default {
name: 'User',
props: ['id'],
// ...
}
```
3. 在组件中直接使用props即可获取路由参数。
```
<template>
<div>User ID: {{ id }}</div>
</template>
<script>
export default {
name: 'User',
props: ['id'],
// ...
}
</script>
```
相关问题
vue3路由传参同时传递多个参数
### 回答1:
在Vue3中,你可以通过以下方式同时传递多个参数:
1. 在路由配置中定义参数:
```
const routes = [
{
path: '/example',
name: 'example',
component: ExampleComponent,
props: { foo: 'foo', bar: 'bar' }
}
]
```
2. 使用动态路由:
```
<router-link :to="{ name: 'example', params: { foo: 'foo', bar: 'bar' } }">Go to Example</router-link>
```
3. 使用查询参数:
```
<router-link :to="{ name: 'example', query: { foo: 'foo', bar: 'bar' } }">Go to Example</router-link>
```
无论哪种方式,你都可以在目标组件中通过 `props` 或 `$route` 来获取传递的参数。例如:
```
export default {
props: ['foo', 'bar'],
mounted() {
console.log(this.foo, this.bar);
console.log(this.$route.params.foo, this.$route.params.bar);
console.log(this.$route.query.foo, this.$route.query.bar);
}
}
```
### 回答2:
在Vue3中,我们可以通过使用路由传参的方式同时传递多个参数。下面是一个简单的示例。
首先,在定义路由时,我们需要设置路由的参数。可以在路由的配置对象中使用`props`属性来设置参数。例如:
```javascript
const routes = [
{
path: '/details',
name: 'details',
component: DetailsComponent,
props: true // 使用props来启用参数传递
}
]
```
然后,在使用路由跳转时,我们可以通过在`$router.push`方法中传递一个对象来传递多个参数。例如:
```javascript
this.$router.push({
name: 'details',
params: {
id: 123,
name: 'Product A',
price: 9.99
}
})
```
最后,在接收参数的组件中,可以通过`props`属性来声明接收的参数,并直接在组件中使用。例如:
```javascript
export default {
props: {
id: {
type: Number,
required: true
},
name: {
type: String,
required: true
},
price: {
type: Number,
required: true
}
},
// ...
}
```
这样,我们就可以同时传递多个参数,并在目标组件中接收并使用这些参数了。
### 回答3:
在Vue3中,可以使用路由的params参数和query参数来传递多个参数。
1. 使用params参数传递多个参数:
```javascript
// 发送路由请求时传递多个参数
this.$router.push({
path: '/example',
params: {
param1: value1,
param2: value2
}
});
// 在目标路由组件中接收参数
value1 = this.$route.params.param1;
value2 = this.$route.params.param2;
```
2. 使用query参数传递多个参数:
```javascript
// 发送路由请求时传递多个参数
this.$router.push({
path: '/example',
query: {
param1: value1,
param2: value2
}
});
// 在目标路由组件中接收参数
value1 = this.$route.query.param1;
value2 = this.$route.query.param2;
```
注意:params参数传递的参数会显示在URL中,例如:`/example/param1/param2`。而query参数传递的参数会在URL之后添加`?`,例如:`/example?param1=value1¶m2=value2`。根据具体需求来选择适合的传参方式。
vue3路由传参如何传递数组
可以使用路由的query参数来传递数组。在路由跳转时,将数组作为参数传递给query,然后在接收方的组件中通过$route.query来获取传递的数组参数。以下是一个示例代码:
1.在路由跳转时传递数组参数:
```javascript
this.$router.push({
path: '/example',
query: {
arr: [1, 2, 3]
}
})
```
2.在接收方的组件中获取传递的数组参数:
```javascript
export default {
mounted() {
const arr = this.$route.query.arr
console.log(arr) // 输出 [1, 2, 3]
}
}
```
阅读全文
相关推荐
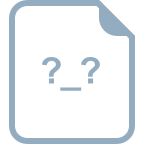














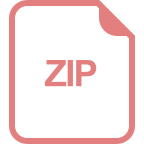