用c++,现要求设计一个表示学生的类,里面有学生的三项成绩:计算机成绩、数学成绩、英语成绩,要求可以求总分、平均分、最高分、最低分,并且可以输出一个学生的完整信息,问:此类该如何设计? 要求:1.根据题目要求设计学生类,除了成绩以外要包含学生ID、姓名、年龄 2.有一个默认无参的构造函数,和一个带参的构造函数,函数的成绩参数是缺省的 3.所有属性必须私有化 4.提供get和set方法用于访问类中的成员 5.设计输出学生信息的方法 6.编写调用测试语句
时间: 2023-05-31 09:07:33 浏览: 56
回答:好的,我了解了您的要求。以下是设计的代码:
```c
#include <iostream>
#include <string>
using namespace std;
class Student {
private:
string id; // 学生ID
string name; // 学生姓名
int age; // 学生年龄
int computer_score; // 计算机成绩
int math_score; // 数学成绩
int english_score; // 英语成绩
public:
// 默认无参构造函数
Student() {
id = "00000";
name = "无名氏";
age = 0;
computer_score = 0;
math_score = 0;
english_score = 0;
}
// 带参构造函数
Student(string id, string name, int age, int computer_score = 0, int math_score = 0, int english_score = 0) {
this->id = id;
this->name = name;
this->age = age;
this->computer_score = computer_score;
this->math_score = math_score;
this->english_score = english_score;
}
// get方法
string get_id() { return id; }
string get_name() { return name; }
int get_age() { return age; }
int get_computer_score() { return computer_score; }
int get_math_score() { return math_score; }
int get_english_score() { return english_score; }
// set方法
void set_id(string id) { this->id = id; }
void set_name(string name) { this->name = name; }
void set_age(int age) { this->age = age; }
void set_computer_score(int computer_score) { this->computer_score = computer_score; }
void set_math_score(int math_score) { this->math_score = math_score; }
void set_english_score(int english_score) { this->english_score = english_score; }
// 总分
int get_total_score() {
return computer_score + math_score + english_score;
}
// 平均分
float get_average_score() {
return (float)get_total_score() / 3.0;
}
// 最高分
int get_highest_score() {
int max_score = computer_score;
if (math_score > max_score) {
max_score = math_score;
}
if (english_score > max_score) {
max_score = english_score;
}
return max_score;
}
// 最低分
int get_lowest_score() {
int min_score = computer_score;
if (math_score < min_score) {
min_score = math_score;
}
if (english_score < min_score) {
min_score = english_score;
}
return min_score;
}
// 输出学生信息
void print_info() {
cout << "学号:" << id << endl;
cout << "姓名:" << name << endl;
cout << "年龄:" << age << endl;
cout << "计算机成绩:" << computer_score << endl;
cout << "数学成绩:" << math_score << endl;
cout << "英语成绩:" << english_score << endl;
cout << "总分:" << get_total_score() << endl;
cout << "平均分:" << get_average_score() << endl;
cout << "最高分:" << get_highest_score() << endl;
cout << "最低分:" << get_lowest_score() << endl;
}
};
int main() {
Student stu("10001", "张三", 18, 88, 90, 85);
stu.print_info();
return 0;
}
```
以上就是对学生类的设计,包括默认构造函数、带参构造函数、私有属性、get和set方法等。最后,输出学生信息并对其访问方法进行测试。
相关推荐
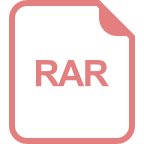
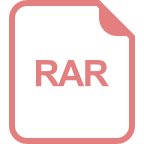
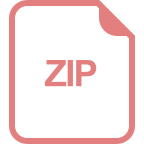














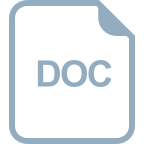