编写一个程序,从data.txt文件读取一批无序数字,按降序排列后再写到新文件dataNew.txt中,c语言
时间: 2024-04-10 08:28:34 浏览: 13
下面是一个示例的程序,可以实现从 `data.txt` 文件读取一批无序数字,按降序排列后再写入到新文件 `dataNew.txt` 中:
```c
#include <stdio.h>
#include <stdlib.h>
// 比较函数,用于 qsort 排序
int compare(const void *a, const void *b) {
return (*(int*)b - *(int*)a);
}
int main() {
// 打开原始文件
FILE *inputFile = fopen("data.txt", "r");
if (inputFile == NULL) {
printf("无法打开原始文件!\n");
return 1;
}
// 读取原始文件中的数字
int numbers[100]; // 假设最多存储100个数字
int count = 0;
while (fscanf(inputFile, "%d", &numbers[count]) != EOF) {
count++;
}
// 关闭原始文件
fclose(inputFile);
// 对数字进行降序排序
qsort(numbers, count, sizeof(int), compare);
// 打开新文件
FILE *outputFile = fopen("dataNew.txt", "w");
if (outputFile == NULL) {
printf("无法打开新文件!\n");
return 1;
}
// 将排序后的数字写入新文件
for (int i = 0; i < count; i++) {
fprintf(outputFile, "%d\n", numbers[i]);
}
// 关闭新文件
fclose(outputFile);
printf("排序完成!请查看 dataNew.txt 文件。\n");
return 0;
}
```
在上面的代码中,我们首先打开原始文件 `data.txt`,然后使用 `fscanf` 函数逐行读取文件中的数字,并将其存储在 `numbers` 数组中。然后,我们使用 `qsort` 函数对数字进行降序排序,使用自定义的 `compare` 函数作为比较函数。接下来,我们打开新文件 `dataNew.txt`,并使用 `fprintf` 函数将排序后的数字逐行写入新文件。最后,我们关闭原始文件和新文件,并输出完成的提示信息。
请确保 `data.txt` 文件存在,并且其中的数字以换行符分隔。程序最多可以处理100个数字,如果超过这个数目,请适当调整 `numbers` 数组的大小。
相关推荐
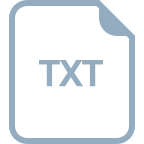














