C语言实现一个绕任意轴任意点的旋转4*4矩阵
时间: 2023-06-01 22:03:25 浏览: 177
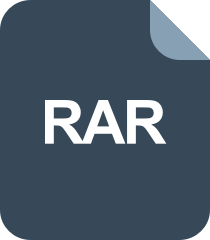
绕任意轴旋转C代码

以下是一个C语言实现绕任意轴任意点的旋转4*4矩阵的示例代码:
```c
#include <math.h>
void rotate_matrix(float* matrix, float angle, float x, float y, float z)
{
float cosA = cos(angle);
float sinA = sin(angle);
float oneMinusCosA = 1.0f - cosA;
// normalize the axis vector
float length = sqrt(x * x + y * y + z * z);
x /= length;
y /= length;
z /= length;
// calculate the rotation matrix
float rotation[16] = {
x * x * oneMinusCosA + cosA,
x * y * oneMinusCosA - z * sinA,
x * z * oneMinusCosA + y * sinA,
0.0f,
y * x * oneMinusCosA + z * sinA,
y * y * oneMinusCosA + cosA,
y * z * oneMinusCosA - x * sinA,
0.0f,
x * z * oneMinusCosA - y * sinA,
y * z * oneMinusCosA + x * sinA,
z * z * oneMinusCosA + cosA,
0.0f,
0.0f,
0.0f,
0.0f,
1.0f
};
// apply the rotation to the matrix
float result[16] = {0};
for (int i = 0; i < 4; i++)
{
for (int j = 0; j < 4; j++)
{
for (int k = 0; k < 4; k++)
{
result[i * 4 + j] += rotation[i * 4 + k] * matrix[k * 4 + j];
}
}
}
// copy the result back to the input matrix
for (int i = 0; i < 16; i++)
{
matrix[i] = result[i];
}
}
```
这个函数接收一个4*4矩阵和一个旋转角度以及一个三维向量作为旋转轴的坐标,然后计算绕该轴旋转后的矩阵,并将结果写回输入矩阵。可以通过多次调用这个函数来实现多次旋转的效果。
阅读全文
相关推荐
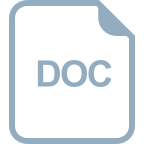
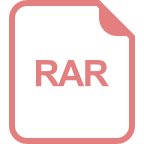
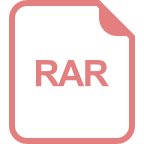
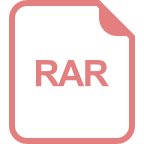
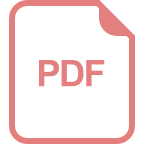
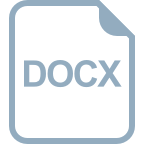
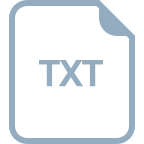
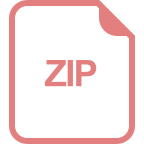
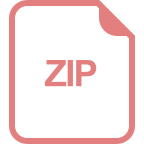
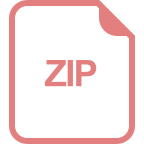
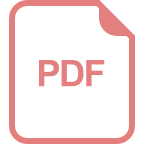
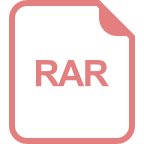