用Python写出机器人搬箱子的代码
时间: 2024-02-13 19:03:55 浏览: 47
好的,让我为你编写一个简单的机器人搬箱子的 Python 代码:
```python
class Robot:
def __init__(self, position):
self.position = position
def move(self, direction):
if direction == 'up':
self.position[1] += 1
elif direction == 'down':
self.position[1] -= 1
elif direction == 'left':
self.position[0] -= 1
elif direction == 'right':
self.position[0] += 1
print(f"Robot moved to position ({self.position[0]}, {self.position[1]})")
class Box:
def __init__(self, position):
self.position = position
def move(self, direction):
if direction == 'up':
self.position[1] += 1
elif direction == 'down':
self.position[1] -= 1
elif direction == 'left':
self.position[0] -= 1
elif direction == 'right':
self.position[0] += 1
print(f"Box moved to position ({self.position[0]}, {self.position[1]})")
robot = Robot([0, 0])
box = Box([1, 1])
# Move the box to the right
box.move('right')
robot.move('right')
# Move the box up
box.move('up')
robot.move('up')
# Move the box to the left
box.move('left')
robot.move('left')
# Move the box down
box.move('down')
robot.move('down')
```
在这个示例中,我们定义了两个类:Robot 和 Box。Robot 和 Box 都有一个位置属性,表示它们的当前位置。它们都有一个 move() 方法,接受一个方向参数('up'、'down'、'left' 或 'right'),并根据方向移动到一个新的位置。在每次移动后,机器人和箱子的位置都会被打印出来。
在主程序中,我们创建了一个 Robot 对象和一个 Box 对象,并将它们放在不同的位置。然后,我们使用 move() 方法将箱子向右移动一个位置,然后让机器人向右移动一个位置,以此类推。
阅读全文
相关推荐
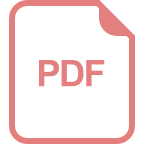
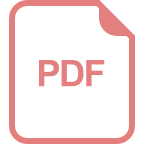
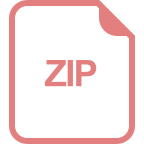
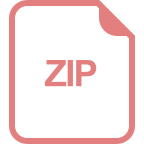

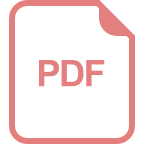
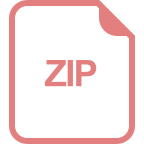
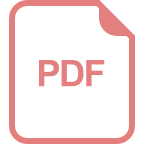
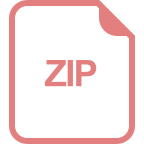
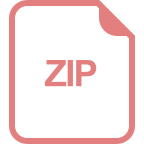
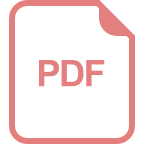
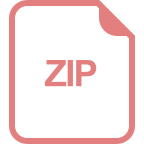
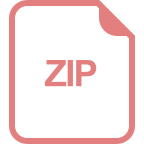