lvgl利用定时器显示DS18B20温度数值代码
时间: 2024-05-07 08:18:31 浏览: 7
以下是一个基于lvgl的温度显示的示例代码,其中使用了定时器更新温度数值:
```c
#include "lvgl/lvgl.h"
#include "lvgl/lvgl_disp.h"
#include "lvgl/lvgl_theme.h"
#include "lvgl/lvgl.h"
#include "lv_drivers/display/fbdev.h"
#include "lv_drivers/indev/evdev.h"
#include <unistd.h>
#include <sys/ioctl.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#define TEMP_SENSOR_PATH "/sys/bus/w1/devices/28-00000xxxxxxx/w1_slave"
#define TEMP_SENSOR_ID "28-00000xxxxxxx"
static lv_obj_t *temp_label;
static void update_temp_value(lv_timer_t *timer)
{
char buf[128];
int fd = open(TEMP_SENSOR_PATH, O_RDONLY);
if (fd < 0) {
lv_label_set_text(temp_label, "Error");
return;
}
int len = read(fd, buf, sizeof(buf));
if (len < 0) {
lv_label_set_text(temp_label, "Error");
close(fd);
return;
}
buf[len] = '\0';
char *p = strstr(buf, "t=");
if (p == NULL) {
lv_label_set_text(temp_label, "Error");
close(fd);
return;
}
int temp = atoi(p+2);
temp = temp / 100.0;
snprintf(buf, sizeof(buf), "Temperature: %d C", temp);
lv_label_set_text(temp_label, buf);
close(fd);
}
int main(int argc, char *argv[])
{
/* Initialize LVGL */
lv_init();
/* Initialize frame buffer device driver */
fbdev_init();
/* Initialize input device driver */
evdev_init();
/* Create a screen */
lv_obj_t *screen = lv_disp_create_screen(NULL, NULL);
/* Create a style */
static lv_style_t style;
lv_style_init(&style);
lv_style_set_text_font(&style, LV_STATE_DEFAULT, &lv_font_roboto_28);
lv_style_set_text_color(&style, LV_STATE_DEFAULT, LV_COLOR_BLACK);
/* Create a label */
temp_label = lv_label_create(screen, NULL);
lv_label_set_text(temp_label, "Loading...");
lv_obj_add_style(temp_label, LV_LABEL_PART_MAIN, &style);
lv_obj_align(temp_label, NULL, LV_ALIGN_CENTER, 0, 0);
/* Create a timer to update temperature value */
lv_timer_create(update_temp_value, 1000, NULL);
/* Start the main loop */
while (1) {
lv_task_handler();
usleep(1000);
}
return 0;
}
```
注意,此代码假设你已经连接了 DS18B20 温度传感器并且已经将其连接到了正确的 GPIO 引脚上。在这个示例中,我们从 `/sys/bus/w1/devices/28-00000xxxxxxx/w1_slave` 文件中读取温度值,其中 `28-00000xxxxxxx` 是你的传感器的 ID,你需要将其替换为你自己传感器的 ID。此外,我们使用定时器每秒钟更新一次温度值,你可以根据自己的需要调整此时间间隔。
相关推荐
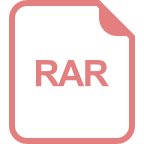
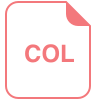
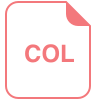
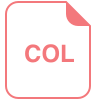
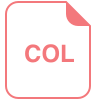
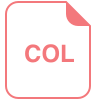









