请用golang写一段链接至“baidu.com”的代码
时间: 2023-09-11 08:05:09 浏览: 97
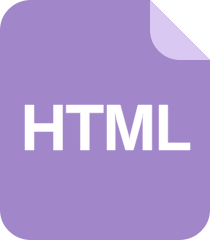
baidu 页面代码
### 回答1:
以下是 Go 语言中连接到 "baidu.com" 的代码片段:
```
package main
import (
"net/http"
"log"
)
func main() {
resp, err := http.Get("http://baidu.com")
if err != nil {
log.Fatalf("http.Get failed: %v", err)
}
defer resp.Body.Close()
}
```
这段代码通过调用 `http.Get` 函数发送了一个 HTTP GET 请求到 "baidu.com",并处理了请求的结果。如果请求失败,则使用 `log.Fatalf` 函数记录错误信息。
### 回答2:
使用Golang编写一个链接到“baidu.com”的代码如下:
```go
package main
import (
"fmt"
"net/http"
)
func main() {
http.HandleFunc("/", indexHandler)
http.ListenAndServe(":8080", nil)
}
func indexHandler(w http.ResponseWriter, r *http.Request) {
http.Redirect(w, r, "https://www.baidu.com", http.StatusFound)
fmt.Println("Redirecting to baidu.com")
}
```
这段代码创建了一个简单的HTTP服务器,当用户访问服务器的根路径时,会进行重定向到百度的网站("https://www.baidu.com")。通过 `http.Redirect` 函数实现重定向,并指定状态码为 `http.StatusFound`(302 Found)。在控制台上,也会输出"Redirecting to baidu.com"。该程序会在本地的8080端口监听HTTP请求。
### 回答3:
在golang中,可以使用`net/http`包来实现http请求和响应。以下是一段使用golang编写的链接至“baidu.com”的代码:
```go
package main
import (
"fmt"
"net/http"
)
func main() {
resp, err := http.Get("http://www.baidu.com")
if err != nil {
fmt.Println("请求出错:", err)
return
}
defer resp.Body.Close()
fmt.Println("响应状态码:", resp.Status)
}
```
在上述代码中,我们使用`http.Get`方法发送HTTP GET请求,传入的参数为要请求的URL。如果请求成功,我们将可以从返回的`resp`变量中获取到响应的相关信息,比如状态码等。
以上代码将输出“响应状态码:200”,表示请求成功。
阅读全文
相关推荐
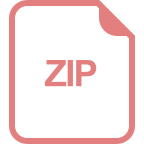
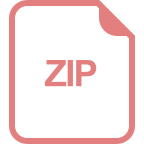
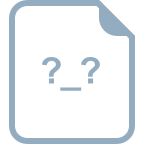
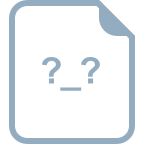
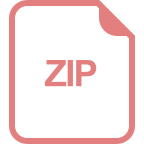
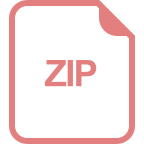
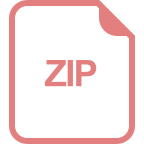
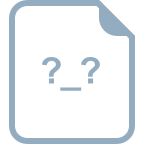
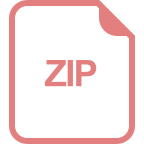