def Print_Txt(screen,font,x,y,text,fcolor=(255,255,255)): Text=font.render(text,True,fcolor) screen.blit(Text,(x,y))
时间: 2024-03-04 22:53:27 浏览: 4
这是一个 Python 函数,它的作用是在屏幕上输出一段文本。具体来说,它接受以下参数:
- `screen`: 表示要输出文本的屏幕。
- `font`: 表示用来渲染文本的字体。
- `x` 和 `y`: 表示文本要输出的位置。
- `text`: 表示要输出的文本内容。
- `fcolor`: 表示文本的颜色,默认为白色。
函数内部通过 `font.render()` 方法将文本渲染成图像,再通过 `screen.blit()` 方法将它绘制到屏幕上。
相关问题
def print_text(font, x, y, text, color=(255,255,255), center=False): imgText = font.render(text, True, color) imgrect = imgText.get_rect() if center: imgrect.center = (x, y) screen.blit(imgText, imgrect) else: screen.blit(imgText, (x,y)) with open("word.txt","r") as f: word = f.readlines() wordList = {} for i in range(len(word)//2): wordList[word[i*2].strip()] = {'Chinese': word[i*2+1].strip(), 'OccurTimes': 0, 'WrongTimes': 0, 'CombineTimes': 0} #复制一个字典用于存储剩余不会的单词 wordListRest = deepcopy(wordList) 解释
这段代码定义了两个函数和两个字典变量。其中,函数print_text用于在屏幕上打印文本,接受5个参数:字体对象、文本的x坐标、y坐标、文本内容、文本颜色和是否居中打印。函数内部使用pygame.font库的render方法生成文本图像,并使用blit方法将图像绘制到屏幕上。
另一个函数是open函数读取文件中的数据。with open()语句中的文件操作会在执行完毕后自动关闭文件,避免出现资源泄露的问题。读取的数据被保存在word变量中。
接下来,代码定义了一个字典变量wordList,用于存储单词的相关信息,包括中文翻译、出现次数、错误次数和组合次数。字典中的键为单词,值为一个包含相关信息的字典。通过循环遍历word变量,将读取到的单词及其翻译存储到wordList中。
最后,代码使用深拷贝函数deepcopy()创建了另一个字典变量wordListRest,用于存储还未掌握的单词。这样可以在不改变原始单词列表的情况下进行单词学习和测试。
帮我把下面代码添加注释class Button: def __init__(self, text='按钮', left=0, top=0, width=90, height=30, color=(200, 200, 200)): self.text = text self.color = color self.rect = pygame.Rect(left, top, width, height) self.font = pygame.font.Font(r"C:\Windows\Fonts\simsun.ttc", 16) self.text_surface = self.font.render(self.text, True, (0, 0, 0)) self.text_rect = self.text_surface.get_rect() # self.text_rect.center = self.rect.center self.active = False self.function = None button_group.append(self) def set_text(self, new_text): self.text = new_text self.text_surface = self.font.render(self.text, True, (0, 0, 0)) self.text_rect = self.text_surface.get_rect() def draw(self, screen): if self.active: pygame.draw.rect(screen, (180, 180, 200), self.rect, 0) else: pygame.draw.rect(screen, self.color, self.rect, 0) self.text_rect.center = self.rect.center screen.blit(self.text_surface, self.text_rect) def get_click(self, event): if event.type == pygame.MOUSEBUTTONDOWN: if event.button == 1: mx, my = event.pos if self.rect.left <= mx <= self.rect.right and self.rect.top <= my <= self.rect.bottom: self.active = True elif event.type == pygame.MOUSEBUTTONUP and self.active: if event.button == 1: mx, my = event.pos if self.rect.left <= mx <= self.rect.right and self.rect.top <= my <= self.rect.bottom: # print(f'{self.text}按钮被点击') if callable(self.function): self.function() self.active = False def click_connect(self, function): self.function = function
以下是代码的注释:
```
class Button:
def __init__(self, text='按钮', left=0, top=0, width=90, height=30, color=(200, 200, 200)):
# 初始化函数,设置按钮的文本、位置、大小和颜色
self.text = text
self.color = color
self.rect = pygame.Rect(left, top, width, height) # 按钮的矩形区域
self.font = pygame.font.Font(r"C:\Windows\Fonts\simsun.ttc", 16) # 按钮文本的字体
self.text_surface = self.font.render(self.text, True, (0, 0, 0)) # 渲染按钮文本
self.text_rect = self.text_surface.get_rect() # 获取按钮文本的矩形区域
# self.text_rect.center = self.rect.center
self.active = False # 按钮是否被点击
self.function = None # 按钮被点击时调用的函数
button_group.append(self) # 将按钮对象添加到按钮组中
def set_text(self, new_text):
# 设置按钮的文本
self.text = new_text
self.text_surface = self.font.render(self.text, True, (0, 0, 0))
self.text_rect = self.text_surface.get_rect()
def draw(self, screen):
# 绘制按钮
if self.active:
pygame.draw.rect(screen, (180, 180, 200), self.rect, 0)
else:
pygame.draw.rect(screen, self.color, self.rect, 0)
self.text_rect.center = self.rect.center
screen.blit(self.text_surface, self.text_rect)
def get_click(self, event):
# 获取鼠标点击事件
if event.type == pygame.MOUSEBUTTONDOWN:
if event.button == 1:
mx, my = event.pos
if self.rect.left <= mx <= self.rect.right and self.rect.top <= my <= self.rect.bottom:
self.active = True
elif event.type == pygame.MOUSEBUTTONUP and self.active:
if event.button == 1:
mx, my = event.pos
if self.rect.left <= mx <= self.rect.right and self.rect.top <= my <= self.rect.bottom:
# print(f'{self.text}按钮被点击')
if callable(self.function):
self.function()
self.active = False
def click_connect(self, function):
# 将按钮与函数连接,当按钮被点击时调用函数
self.function = function
```
相关推荐
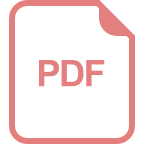
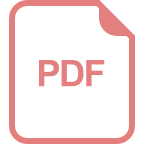
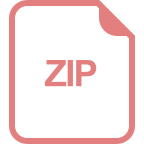








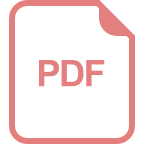
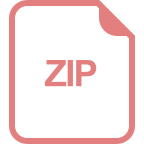
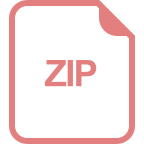
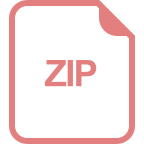
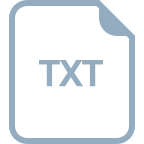