vue实现有权限的账号可以对表格中的3种资源类型按钮(数据库类型、接口类型、文件类型)操作,如选中按钮、取消选中按钮操作;没有权限的账号则不可操作资源类型按钮,并且表格中的资源类型数据改成从后端接口获取返回页面展示
时间: 2024-03-05 08:49:22 浏览: 22
好的,针对您的问题,我可以给出以下的解答:
1. 针对权限问题,可以在 Vue 中使用组件的指令 v-if 或 v-show 来实现。在渲染表格时,可以根据当前用户的权限来判断是否显示资源类型按钮。具体实现可参考如下代码:
```html
<template>
<div>
<table>
<thead>
<tr>
<th>资源名称</th>
<th v-if="hasPermission">资源类型</th>
</tr>
</thead>
<tbody>
<tr v-for="item in tableData" :key="item.id">
<td>{{ item.name }}</td>
<td v-if="hasPermission">
<div>
<label>
<input type="checkbox" v-model="item.databaseType" />
数据库类型
</label>
<label>
<input type="checkbox" v-model="item.interfaceType" />
接口类型
</label>
<label>
<input type="checkbox" v-model="item.fileType" />
文件类型
</label>
</div>
</td>
</tr>
</tbody>
</table>
</div>
</template>
<script>
export default {
data() {
return {
tableData: [],
hasPermission: false, // 根据当前用户的权限获取
};
},
methods: {
changeResourceType(id) {
// 修改资源类型逻辑
},
},
};
</script>
```
2. 针对表格中的资源类型数据从后端接口获取,可以在 Vue 的生命周期函数 created 或 mounted 中调用接口获取数据并保存到组件的 data 中,然后在页面中渲染。具体实现可参考如下代码:
```html
<template>
<div>
<table>
<thead>
<tr>
<th>资源名称</th>
<th v-if="hasPermission">资源类型</th>
</tr>
</thead>
<tbody>
<tr v-for="item in tableData" :key="item.id">
<td>{{ item.name }}</td>
<td v-if="hasPermission">
<div>
<label>
<input type="checkbox" v-model="item.databaseType" />
数据库类型
</label>
<label>
<input type="checkbox" v-model="item.interfaceType" />
接口类型
</label>
<label>
<input type="checkbox" v-model="item.fileType" />
文件类型
</label>
</div>
</td>
</tr>
</tbody>
</table>
</div>
</template>
<script>
export default {
data() {
return {
tableData: [],
hasPermission: false, // 根据当前用户的权限获取
};
},
created() {
// 调用接口获取表格数据
this.$axios.get('/api/tableData').then((res) => {
this.tableData = res.data;
});
},
methods: {
changeResourceType(id) {
// 修改资源类型逻辑
},
},
};
</script>
```
以上就是我对您问题的回答,希望能对您有所帮助。
相关推荐
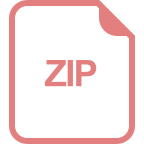
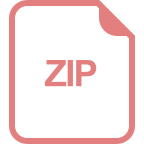
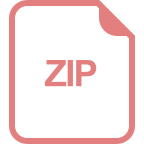














