Android bitmap 保存bmp
时间: 2023-08-07 19:43:06 浏览: 105
要将 Android Bitmap 保存为 BMP 格式,可以使用以下步骤:
1. 创建一个 BITMAPFILEHEADER 结构体,用于保存 BMP 文件头信息。
2. 创建一个 BITMAPINFOHEADER 结构体,用于保存位图信息头。
3. 计算位图像素数据的大小,用于分配内存。
4. 将 Android Bitmap 转换为 RGB 格式的位图像素数据。
5. 将位图像素数据按照 BMP 文件格式存储到内存中。
6. 将 BITMAPFILEHEADER 和 BITMAPINFOHEADER 写入 BMP 文件开头。
7. 将位图像素数据写入 BMP 文件中。
以下是一个示例代码,用于将 Android Bitmap 保存为 BMP 格式:
```java
import android.graphics.Bitmap;
import android.graphics.Color;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.nio.ByteBuffer;
import java.nio.ByteOrder;
public class BitmapUtils {
// BMP 文件头
private static class BITMAPFILEHEADER {
public char bfType[] = {'B', 'M'};
public int bfSize;
public short bfReserved1;
public short bfReserved2;
public int bfOffBits;
}
// BMP 位图信息头
private static class BITMAPINFOHEADER {
public int biSize;
public int biWidth;
public int biHeight;
public short biPlanes;
public short biBitCount;
public int biCompression;
public int biSizeImage;
public int biXPelsPerMeter;
public int biYPelsPerMeter;
public int biClrUsed;
public int biClrImportant;
}
// 将 Android Bitmap 保存为 BMP 格式
public static boolean saveBitmapAsBmp(Bitmap bitmap, String filename) {
// 获取位图信息
int width = bitmap.getWidth();
int height = bitmap.getHeight();
// 计算位图像素数据大小
int data_size = width * height * 3;
// 创建 BMP 文件头
BITMAPFILEHEADER file_header = new BITMAPFILEHEADER();
file_header.bfSize = 14 + 40 + data_size;
file_header.bfReserved1 = 0;
file_header.bfReserved2 = 0;
file_header.bfOffBits = 14 + 40;
// 创建 BMP 位图信息头
BITMAPINFOHEADER info_header = new BITMAPINFOHEADER();
info_header.biSize = 40;
info_header.biWidth = width;
info_header.biHeight = height;
info_header.biPlanes = 1;
info_header.biBitCount = 24;
info_header.biCompression = 0;
info_header.biSizeImage = data_size;
info_header.biXPelsPerMeter = 0;
info_header.biYPelsPerMeter = 0;
info_header.biClrUsed = 0;
info_header.biClrImportant = 0;
// 将 Android Bitmap 转换为 RGB 格式的位图像素数据
int[] pixels = new int[width * height];
bitmap.getPixels(pixels, 0, width, 0, 0, width, height);
byte[] data = new byte[data_size];
int i = 0;
for (int y = height - 1; y >= 0; y--) {
for (int x = 0; x < width; x++) {
int pixel = pixels[y * width + x];
data[i++] = (byte) Color.blue(pixel);
data[i++] = (byte) Color.green(pixel);
data[i++] = (byte) Color.red(pixel);
}
}
// 写入 BMP 文件
try {
FileOutputStream fos = new FileOutputStream(new File(filename));
ByteBuffer buffer = ByteBuffer.allocate(14 + 40 + data_size);
buffer.order(ByteOrder.LITTLE_ENDIAN);
buffer.putChar('B');
buffer.putChar('M');
buffer.putInt(file_header.bfSize);
buffer.putShort(file_header.bfReserved1);
buffer.putShort(file_header.bfReserved2);
buffer.putInt(file_header.bfOffBits);
buffer.putInt(info_header.biSize);
buffer.putInt(info_header.biWidth);
buffer.putInt(info_header.biHeight);
buffer.putShort(info_header.biPlanes);
buffer.putShort(info_header.biBitCount);
buffer.putInt(info_header.biCompression);
buffer.putInt(info_header.biSizeImage);
buffer.putInt(info_header.biXPelsPerMeter);
buffer.putInt(info_header.biYPelsPerMeter);
buffer.putInt(info_header.biClrUsed);
buffer.putInt(info_header.biClrImportant);
buffer.put(data);
fos.write(buffer.array());
fos.close();
return true;
} catch (IOException e) {
e.printStackTrace();
return false;
}
}
}
```
这个函数需要传入一个 Android Bitmap 对象和一个保存文件名,函数会将 Android Bitmap 保存为 BMP 格式,并返回一个布尔值表示保存是否成功。
相关推荐
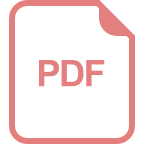













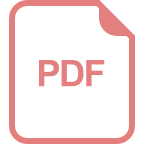
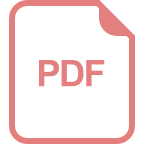