用c语言创建5个进程,其中两个进程为生产者进程,3个进程为消费者进程。一个生产者进程试图不断地在一个缓冲中写入大写字母,另一个生产者进程试图不断地在缓冲中写入小写字母。3个消费者不断地从缓冲中读取一个字符并输出。为了使得程序的输出易于看到结果,分别在生产者和消费者进程的合适的位置加入一些随机睡眠时间。用信号量作为同步互斥机制
时间: 2024-03-11 19:51:24 浏览: 4
好的,这是一个经典的生产者消费者问题,可以用信号量来实现进程间的同步和互斥。下面是一个示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <pthread.h>
#include <semaphore.h>
#define BUFFER_SIZE 5
#define MAX_COUNT 10
char buffer[BUFFER_SIZE];
int in = 0, out = 0, count = 0;
sem_t empty, full, mutex;
void *producer_uppercase(void *arg) {
int i;
char c;
for (i = 0; i < MAX_COUNT; i++) {
c = 'A' + rand() % 26;
sem_wait(&empty);
sem_wait(&mutex);
buffer[in] = c;
in = (in + 1) % BUFFER_SIZE;
count++;
printf("Producer(uppercase) produce %c, count = %d\n", c, count);
sem_post(&mutex);
sem_post(&full);
sleep(rand() % 3);
}
pthread_exit(NULL);
}
void *producer_lowercase(void *arg) {
int i;
char c;
for (i = 0; i < MAX_COUNT; i++) {
c = 'a' + rand() % 26;
sem_wait(&empty);
sem_wait(&mutex);
buffer[in] = c;
in = (in + 1) % BUFFER_SIZE;
count++;
printf("Producer(lowercase) produce %c, count = %d\n", c, count);
sem_post(&mutex);
sem_post(&full);
sleep(rand() % 3);
}
pthread_exit(NULL);
}
void *consumer(void *arg) {
int i;
char c;
for (i = 0; i < MAX_COUNT * 2; i++) {
sem_wait(&full);
sem_wait(&mutex);
c = buffer[out];
out = (out + 1) % BUFFER_SIZE;
count--;
printf("Consumer consume %c, count = %d\n", c, count);
sem_post(&mutex);
sem_post(&empty);
sleep(rand() % 3);
}
pthread_exit(NULL);
}
int main(int argc, char *argv[]) {
pthread_t tid[5];
sem_init(&empty, 0, BUFFER_SIZE);
sem_init(&full, 0, 0);
sem_init(&mutex, 0, 1);
pthread_create(&tid[0], NULL, producer_uppercase, NULL);
pthread_create(&tid[1], NULL, producer_lowercase, NULL);
pthread_create(&tid[2], NULL, consumer, NULL);
pthread_create(&tid[3], NULL, consumer, NULL);
pthread_create(&tid[4], NULL, consumer, NULL);
pthread_join(tid[0], NULL);
pthread_join(tid[1], NULL);
pthread_join(tid[2], NULL);
pthread_join(tid[3], NULL);
pthread_join(tid[4], NULL);
sem_destroy(&empty);
sem_destroy(&full);
sem_destroy(&mutex);
return 0;
}
```
这个程序会创建5个线程,其中两个线程为生产者线程,三个线程为消费者线程。使用了三个信号量`empty`、`full`、`mutex`,分别表示缓冲区空余的大小、缓冲区已占用的大小、互斥访问缓冲区的锁。在生产者线程中,先用`sem_wait`操作等待`empty`信号量,表示缓冲区中有空位可以写入;再用`sem_wait`操作等待`mutex`信号量,表示要访问缓冲区了,需要先获取锁;然后将数据写入缓冲区,更新计数器,并输出相应的信息;最后用`sem_post`操作释放锁和`full`信号量,表示缓冲区已经有数据可供读取。在消费者线程中,先用`sem_wait`操作等待`full`信号量,表示缓冲区中有数据可读;再用`sem_wait`操作等待`mutex`信号量,表示要访问缓冲区了,需要先获取锁;然后将数据从缓冲区中读取,更新计数器,并输出相应的信息;最后用`sem_post`操作释放锁和`empty`信号量,表示缓冲区已经有空位可供写入。在两个生产者线程和三个消费者线程中,都加入了`sleep`操作,模拟了一定的随机时间,使得输出结果更加随机和真实。
相关推荐
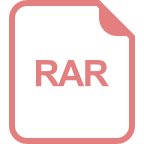
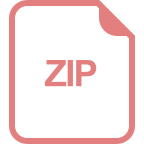
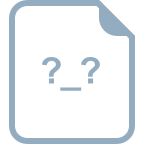















