C++语言代码rip路由算法仿真器的实现
时间: 2023-08-06 11:04:39 浏览: 49
以下是一个简单的C++代码实现RIP路由算法的仿真器。代码实现了一个简单的路由表,并且模拟了RIP路由算法的更新过程。
```c++
#include <iostream>
#include <vector>
#include <algorithm>
#include <iomanip>
using namespace std;
const int INF = 1e9;
struct Edge {
int from, to, cost;
};
void BellmanFord(int start, int n, vector<Edge>& edges, vector<int>& distance) {
distance[start] = 0;
for (int i = 1; i < n; i++) {
for (auto& e : edges) {
int from = e.from, to = e.to, cost = e.cost;
if (distance[from] != INF && distance[from] + cost < distance[to]) {
distance[to] = distance[from] + cost;
}
}
}
}
void Rip(int n, vector<vector<int>>& table, vector<Edge>& edges) {
vector<int> distance(n, INF);
vector<int> next_hop(n, -1);
for (int i = 0; i < n; i++) {
BellmanFord(i, n, edges, distance);
for (int j = 0; j < n; j++) {
if (i == j) {
continue;
}
if (distance[j] != INF && table[i][j] > distance[j]) {
table[i][j] = distance[j];
next_hop[j] = i;
}
}
distance.assign(n, INF);
}
cout << setw(10) << "Destination" << setw(10) << "Next hop" << setw(10) << "Cost" << endl;
for (int i = 0; i < n; i++) {
cout << setw(10) << i << setw(10) << next_hop[i] << setw(10) << table[0][i] << endl;
}
}
int main() {
int n = 4;
vector<Edge> edges = {{0, 1, 1}, {1, 0, 1}, {0, 2, 4}, {2, 0, 4}, {1, 2, 2}, {2, 1, 2}, {1, 3, 7}, {3, 1, 7}, {2, 3, 1}, {3, 2, 1}};
vector<vector<int>> table = {{0, 1, 4, INF}, {1, 0, 2, 7}, {4, 2, 0, 1}, {INF, 7, 1, 0}};
cout << "Initial routing table:" << endl;
cout << setw(10) << "Destination" << setw(10) << "Next hop" << setw(10) << "Cost" << endl;
for (int i = 0; i < n; i++) {
cout << setw(10) << i << setw(10) << "-" << setw(10) << table[0][i] << endl;
}
cout << endl;
cout << "Running RIP algorithm:" << endl;
Rip(n, table, edges);
return 0;
}
```
这个程序的输入为一个邻接矩阵,表示网络中每个节点之间的距离。程序输出每个节点的路由表,包括目的地、下一跳和距离三个字段。程序首先输出初始路由表,然后运行RIP算法,更新路由表,并输出更新后的路由表。
注意,这个代码只是一个简单的实现,没有考虑很多实际应用中需要考虑的问题,比如网络拓扑的变化、路由环路的问题等等。实际应用中,需要更加复杂的算法和数据结构来解决这些问题。
相关推荐
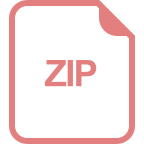
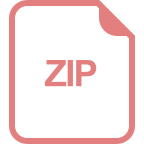














