STM32G071RBT6 FLASH 读写数据示例
时间: 2024-06-17 11:03:01 浏览: 18
STM32G071RBT6是一款Cortex-M0+内核的微控制器,它集成了128 KB Flash存储器和36 KB SRAM存储器。下面是一个简单的STM32G071RBT6 FLASH 读写数据示例。
首先,需要在代码中定义Flash的起始地址,例如:
```
#define FLASH_START_ADDRESS ((uint32_t)0x08000000) // Flash起始地址为0x08000000
```
接下来,我们可以使用HAL库提供的函数来进行Flash的读写操作。下面是一个示例程序,可以将一个字符串写入Flash,并从Flash中读取该字符串:
```
#include "main.h"
#include <string.h>
#define FLASH_START_ADDRESS ((uint32_t)0x08000000)
void write_flash(uint32_t address, uint32_t data);
uint32_t read_flash(uint32_t address);
int main(void)
{
char string_to_write[] = "Hello, world!";
uint32_t address_to_write = FLASH_START_ADDRESS + 0x100; // 从0x08000100地址开始写入数据
// 将字符串写入Flash
HAL_FLASH_Unlock();
for (int i = 0; i < strlen(string_to_write); i++) {
write_flash(address_to_write + i, string_to_write[i]);
}
HAL_FLASH_Lock();
// 从Flash中读取字符串
char string_read[strlen(string_to_write) + 1];
for (int i = 0; i < strlen(string_to_write); i++) {
string_read[i] = read_flash(address_to_write + i);
}
string_read[strlen(string_to_write)] = '\0'; // 将读取的字符串末尾添加'\0'字符,以表示字符串结束
while (1);
}
// 写入Flash
void write_flash(uint32_t address, uint32_t data)
{
HAL_StatusTypeDef status;
status = HAL_FLASH_Program(FLASH_TYPEPROGRAM_WORD, address, data);
if (status != HAL_OK) {
// 写入失败
while (1);
}
}
// 从Flash中读取数据
uint32_t read_flash(uint32_t address)
{
return (*(__IO uint32_t*)address);
}
```
上述代码中,write_flash函数将一个字节写入Flash,read_flash函数从Flash中读取一个字节。在main函数中,我们将字符串"Hello, world!"写入Flash的地址0x08000100开始的位置,并从该位置读取该字符串。注意,在写入或读取Flash时,需要先使用HAL_FLASH_Unlock函数解锁Flash,操作完成后再使用HAL_FLASH_Lock函数锁定Flash。
相关推荐
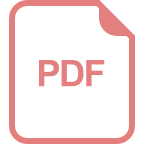














