在table view某一列实现Button
时间: 2024-02-26 20:56:28 浏览: 148
要在table view的某一列实现button,可以通过以下步骤实现:
1. 在table view的数据源中添加一个按钮的属性,用来记录当前cell需要展示的按钮状态。
2. 在table view的代理方法中,处理cell的按钮状态。可以通过为cell添加一个UIButton的子视图,并设置按钮的标题、样式等来实现。
3. 在点击按钮时,更新数据源中的按钮状态,并重新加载对应的cell。
以下是一个简单的示例代码:
```
// 数据源中添加按钮的状态属性
enum ButtonState {
case normal
case selected
}
struct Item {
var title: String
var buttonState: ButtonState
}
var items: [Item] = [
Item(title: "item 1", buttonState: .normal),
Item(title: "item 2", buttonState: .normal),
Item(title: "item 3", buttonState: .normal),
]
// 处理cell的按钮状态
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "Cell", for: indexPath)
let item = items[indexPath.row]
cell.textLabel?.text = item.title
// 添加按钮
let button = UIButton(type: .system)
button.frame = CGRect(x: cell.contentView.bounds.width - 80, y: 0, width: 80, height: cell.contentView.bounds.height)
button.titleLabel?.font = UIFont.systemFont(ofSize: 16)
button.addTarget(self, action: #selector(buttonTapped(_:)), for: .touchUpInside)
cell.contentView.addSubview(button)
// 设置按钮的标题和样式
switch item.buttonState {
case .normal:
button.setTitle("Normal", for: .normal)
button.setTitleColor(.blue, for: .normal)
case .selected:
button.setTitle("Selected", for: .normal)
button.setTitleColor(.red, for: .normal)
}
return cell
}
// 点击按钮时更新按钮的状态
@objc func buttonTapped(_ sender: UIButton) {
guard let cell = sender.superview?.superview as? UITableViewCell,
let indexPath = tableView.indexPath(for: cell) else {
return
}
switch items[indexPath.row].buttonState {
case .normal:
items[indexPath.row].buttonState = .selected
case .selected:
items[indexPath.row].buttonState = .normal
}
tableView.reloadData()
}
```
在以上示例代码中,我们通过ButtonState枚举来记录按钮的状态。在处理cell的按钮状态时,我们添加了一个UIButton的子视图,并根据按钮的状态设置按钮的标题和样式。在点击按钮时,我们根据按钮所在的cell的indexPath更新按钮的状态,并重新加载对应的cell。需要注意的是,由于UIButton添加到cell.contentView上,因此需要调用sender.superview?.superview来获取cell的引用。
阅读全文
相关推荐
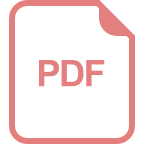
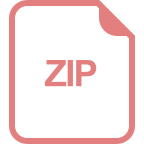
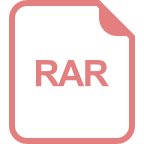
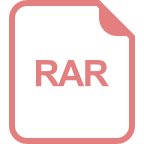
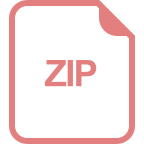
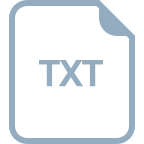
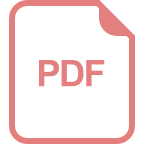
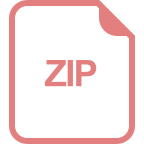
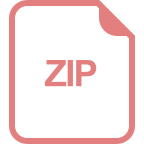
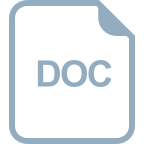
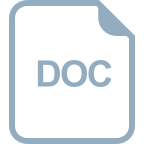




