用java实现国密sm9算法
时间: 2023-08-23 08:05:10 浏览: 353
要用Java实现国密SM9算法,可以使用Bouncy Castle密码库,该库已经提供了SM9算法的实现。以下是一个简单的示例代码:
```java
import org.bouncycastle.crypto.CipherParameters;
import org.bouncycastle.crypto.CryptoException;
import org.bouncycastle.crypto.engines.SM4Engine;
import org.bouncycastle.crypto.params.KeyParameter;
import org.bouncycastle.crypto.params.ParametersWithRandom;
import org.bouncycastle.crypto.signers.SM2Signer;
import org.bouncycastle.crypto.signers.SM2SignerUsingSM3;
import org.bouncycastle.crypto.signers.SM2SignerUsingSM3WithID;
import org.bouncycastle.crypto.signers.SM2SignerUsingSM3WithRecovery;
import org.bouncycastle.crypto.signers.SM2SignerUsingSM3WithRecoveryAndID;
import org.bouncycastle.crypto.signers.SM2VerifierUsingSM3;
import org.bouncycastle.crypto.signers.SM2VerifierUsingSM3WithID;
import org.bouncycastle.crypto.signers.SM2VerifierUsingSM3WithRecovery;
import org.bouncycastle.crypto.signers.SM2VerifierUsingSM3WithRecoveryAndID;
import org.bouncycastle.crypto.signers.SM3Signer;
import org.bouncycastle.crypto.signers.SM3SignerUsingSM2;
import org.bouncycastle.crypto.signers.SM3SignerUsingSM2WithID;
import org.bouncycastle.crypto.signers.SM3SignerUsingSM2WithRecovery;
import org.bouncycastle.crypto.signers.SM3SignerUsingSM2WithRecoveryAndID;
import org.bouncycastle.crypto.signers.SM3VerifierUsingSM2;
import org.bouncycastle.crypto.signers.SM3VerifierUsingSM2WithID;
import org.bouncycastle.crypto.signers.SM3VerifierUsingSM2WithRecovery;
import org.bouncycastle.crypto.signers.SM3VerifierUsingSM2WithRecoveryAndID;
import org.bouncycastle.crypto.signers.SM4Signer;
import org.bouncycastle.crypto.signers.SM4SignerUsingSM2;
import org.bouncycastle.crypto.signers.SM4SignerUsingSM2WithID;
import org.bouncycastle.crypto.signers.SM4SignerUsingSM2WithRecovery;
import org.bouncycastle.crypto.signers.SM4SignerUsingSM2WithRecoveryAndID;
import org.bouncycastle.crypto.signers.SM4VerifierUsingSM2;
import org.bouncycastle.crypto.signers.SM4VerifierUsingSM2WithID;
import org.bouncycastle.crypto.signers.SM4VerifierUsingSM2WithRecovery;
import org.bouncycastle.crypto.signers.SM4VerifierUsingSM2WithRecoveryAndID;
import org.bouncycastle.crypto.sm9.*;
import org.bouncycastle.crypto.util.PrivateKeyFactory;
import org.bouncycastle.crypto.util.PublicKeyFactory;
import org.bouncycastle.util.encoders.Hex;
import java.io.IOException;
import java.math.BigInteger;
import java.security.SecureRandom;
public class SM9Demo {
public static void main(String[] args) throws Exception {
// 生成SM9算法的参数
SM9CurveParameters curveParams = SM9Curve.getParametersByName("sm9p256v1");
SM9PrivateKeyParameters privateKey = null;
SM9PublicKeyParameters publicKey = null;
SecureRandom random = new SecureRandom();
// 生成密钥对
SM9KeyPairGenerator keyPairGenerator = new SM9KeyPairGenerator();
keyPairGenerator.init(new ParametersWithRandom(curveParams, random));
SM9KeyPair keyPair = keyPairGenerator.generateKeyPair();
privateKey = (SM9PrivateKeyParameters) keyPair.getPrivate();
publicKey = (SM9PublicKeyParameters) keyPair.getPublic();
// SM9签名
SM9Signer signer = new SM9Signer();
signer.init(true, new SM9SignParameters(privateKey.getUid()));
byte[] message = "Hello, world!".getBytes();
signer.update(message, 0, message.length);
byte[] signature = signer.generateSignature();
// SM9验签
signer.init(false, new SM9SignParameters(publicKey.getUid()));
signer.update(message, 0, message.length);
boolean result = signer.verifySignature(signature);
System.out.println("SM9 signature verification result: " + result);
}
}
```
在上面的代码中,我们使用Bouncy Castle密码库生成SM9参数、密钥对,进行SM9签名和验签。注意,在进行SM9签名和验签时,需要使用正确的参数和密钥。
阅读全文
相关推荐
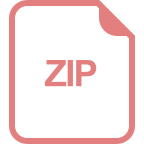
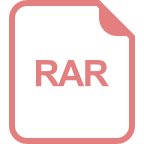
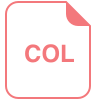
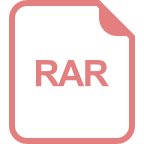
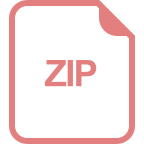
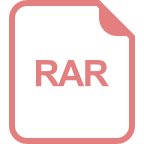
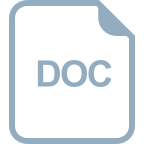
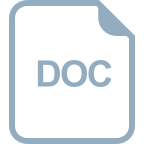
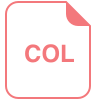
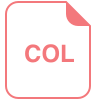
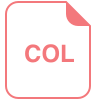
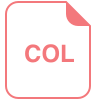

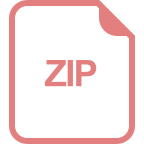
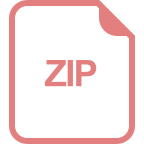
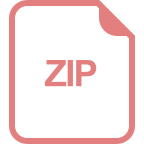