C语言写一个小车的惯性导航代码
时间: 2024-05-04 07:19:32 浏览: 8
惯性导航是一种基于车辆惯性力量和姿态传感器数据的导航方式,主要使用陀螺仪和加速度计来测量车辆的姿态和加速度,然后通过数学模型计算车辆的位置、速度和方向。以下是一个简单的使用C语言实现惯性导航的代码示例:
```c
#include <stdio.h>
#include <math.h>
#define PI 3.14159265359
typedef struct {
double x;
double y;
double z;
} Vector;
typedef struct {
Vector position;
Vector velocity;
Vector acceleration;
Vector orientation;
Vector angularVelocity;
Vector angularAcceleration;
} CarState;
int main() {
CarState car = { {0, 0, 0}, {0, 0, 0}, {0, 0, 0}, {0, 0, 0}, {0, 0, 0}, {0, 0, 0} };
double timeStep = 0.1; // 时间步长
double speed = 10; // 初始速度
double heading = 0; // 初始航向角度
double pitch = 0; // 初始俯仰角度
double roll = 0; // 初始翻滚角度
double gyroX = 0; // 陀螺仪X轴输出
double gyroY = 0; // 陀螺仪Y轴输出
double gyroZ = 0; // 陀螺仪Z轴输出
double accX = 0; // 加速度计X轴输出
double accY = 0; // 加速度计Y轴输出
double accZ = 0; // 加速度计Z轴输出
double mass = 1000; // 小车质量
double wheelBase = 2.5; // 小车轴距
double wheelTrack = 1.5; // 小车轮距
double wheelRadius = 0.25; // 小车轮半径
double mu = 0.1; // 摩擦系数
double steeringAngle = 0; // 方向盘转角
double throttle = 0; // 油门开度
while (1) {
// 计算小车姿态
car.orientation.x += car.angularVelocity.x * timeStep;
car.orientation.y += car.angularVelocity.y * timeStep;
car.orientation.z += car.angularVelocity.z * timeStep;
// 计算小车速度
car.velocity.x += car.acceleration.x * timeStep;
car.velocity.y += car.acceleration.y * timeStep;
car.velocity.z += car.acceleration.z * timeStep;
// 计算小车位置
car.position.x += car.velocity.x * timeStep;
car.position.y += car.velocity.y * timeStep;
car.position.z += car.velocity.z * timeStep;
// 读取传感器数据
gyroX = 0; // TODO: 读取陀螺仪X轴数据
gyroY = 0; // TODO: 读取陀螺仪Y轴数据
gyroZ = 0; // TODO: 读取陀螺仪Z轴数据
accX = 0; // TODO: 读取加速度计X轴数据
accY = 0; // TODO: 读取加速度计Y轴数据
accZ = 0; // TODO: 读取加速度计Z轴数据
// 计算小车加速度
car.acceleration.x = accX;
car.acceleration.y = accY;
car.acceleration.z = accZ;
// 计算小车角速度
car.angularVelocity.x = gyroX;
car.angularVelocity.y = gyroY;
car.angularVelocity.z = gyroZ;
// 计算小车角加速度
car.angularAcceleration.x = (steeringAngle / wheelBase) * speed * cos(pitch) + (1 / mass) * (wheelTrack / 2) * (throttle - mu * mass * 9.8 * sin(pitch)) * cos(heading);
car.angularAcceleration.y = (steeringAngle / wheelBase) * speed * sin(pitch) + (1 / mass) * (wheelTrack / 2) * (throttle - mu * mass * 9.8 * sin(pitch)) * sin(heading);
car.angularAcceleration.z = (1 / mass) * (wheelTrack / 2) * (throttle - mu * mass * 9.8 * sin(pitch)) * sin(steeringAngle);
// 更新小车状态
speed += car.acceleration.z * timeStep;
heading += (speed / wheelBase) * tan(steeringAngle) * timeStep;
pitch += car.angularVelocity.x * timeStep;
roll += car.angularVelocity.y * timeStep;
// 输出小车状态
printf("Position: (%lf, %lf, %lf)\n", car.position.x, car.position.y, car.position.z);
printf("Velocity: (%lf, %lf, %lf)\n", car.velocity.x, car.velocity.y, car.velocity.z);
printf("Acceleration: (%lf, %lf, %lf)\n", car.acceleration.x, car.acceleration.y, car.acceleration.z);
printf("Orientation: (%lf, %lf, %lf)\n", car.orientation.x, car.orientation.y, car.orientation.z);
printf("Angular Velocity: (%lf, %lf, %lf)\n", car.angularVelocity.x, car.angularVelocity.y, car.angularVelocity.z);
printf("Angular Acceleration: (%lf, %lf, %lf)\n", car.angularAcceleration.x, car.angularAcceleration.y, car.angularAcceleration.z);
// 等待下一个时间步长
// TODO: 通过延时函数等待下一个时间步长
}
return 0;
}
```
这只是一个简单的示例代码,实际应用中还需要根据具体情况进行调整和优化。
相关推荐
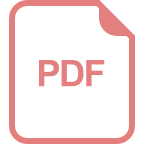
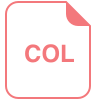
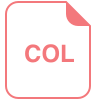
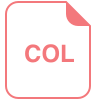
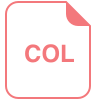
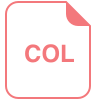










