超类 Circle派生了一个称为 Cylinder 的子类,如下方类图所示。研究子类 Cylinder 如何调用超类 Circle 的构造函数,并从超类继承和使用方法 getArea()。Circle 属性 radius:圆半径。 color:圆颜色。 构造方法 Circle():初始化属性。 public Circle(double radius)。 public Circle(double radius,String color)。 方法 两个属性的set和get方法。 getArea():返回圆的面积。 toString(): 返回Circle[radius=xxx,color=xxx]。 Cylinder 属性 height:圆柱的高。 构造方法 Cylinder():初始化属性。 Cylinder(double height)。 Cylinder(double radius,double height)。 Cylinder(double radius, double height,String color)。 方法 属性height的set和get方法。 getVolume():返回圆柱体的体积(圆柱体体积计算公式为底面积x高)。 题目要求 根据题目描述和类图,完成圆和圆柱类。 注:在main方法当中测试类中的属性、方法、构造器。(用java语言)
时间: 2023-11-27 19:52:11 浏览: 45
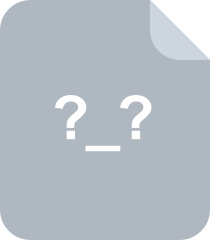
设计基类点类(Point)、直接派生类圆类(Circle)、间接派生类圆柱体类(Cylinder),将求面积的函数(area)声明成虚函数,并在主函数实现虚函数
以下是Circle和Cylinder类的实现:
```java
// Circle类
public class Circle {
private double radius;
private String color;
public Circle() {
this.radius = 1.0;
this.color = "red";
}
public Circle(double radius) {
this.radius = radius;
this.color = "red";
}
public Circle(double radius, String color) {
this.radius = radius;
this.color = color;
}
public double getRadius() {
return this.radius;
}
public void setRadius(double radius) {
this.radius = radius;
}
public String getColor() {
return this.color;
}
public void setColor(String color) {
this.color = color;
}
public double getArea() {
return Math.PI * radius * radius;
}
public String toString() {
return "Circle[radius=" + radius + ",color=" + color + "]";
}
}
// Cylinder类
public class Cylinder extends Circle {
private double height;
public Cylinder() {
super();
this.height = 1.0;
}
public Cylinder(double height) {
super();
this.height = height;
}
public Cylinder(double radius, double height) {
super(radius);
this.height = height;
}
public Cylinder(double radius, double height, String color) {
super(radius, color);
this.height = height;
}
public double getHeight() {
return this.height;
}
public void setHeight(double height) {
this.height = height;
}
public double getVolume() {
return this.getArea() * this.height;
}
public String toString() {
return "Cylinder[height=" + height + "," + super.toString() + "]";
}
}
```
在main方法中,我们可以使用以下代码来测试类的属性、方法和构造器:
```java
public static void main(String[] args) {
Circle c1 = new Circle();
System.out.println("Radius is " + c1.getRadius()
+ ", Color is " + c1.getColor()
+ ", Area is " + c1.getArea());
Circle c2 = new Circle(2.0);
System.out.println("Radius is " + c2.getRadius()
+ ", Color is " + c2.getColor()
+ ", Area is " + c2.getArea());
Cylinder cy1 = new Cylinder();
System.out.println("Radius is " + cy1.getRadius()
+ ", Height is " + cy1.getHeight()
+ ", Color is " + cy1.getColor()
+ ", Volume is " + cy1.getVolume());
Cylinder cy2 = new Cylinder(2.0, 3.0);
System.out.println("Radius is " + cy2.getRadius()
+ ", Height is " + cy2.getHeight()
+ ", Color is " + cy2.getColor()
+ ", Volume is " + cy2.getVolume());
}
```
阅读全文
相关推荐
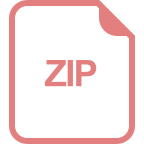



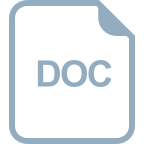
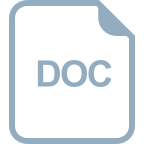
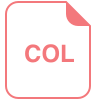


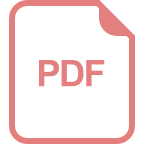
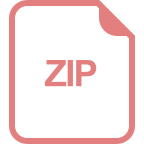
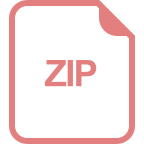
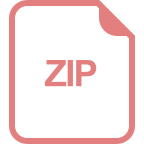
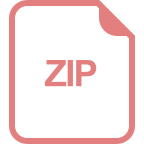
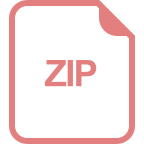
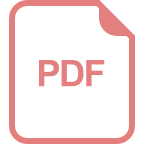
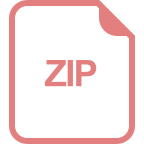
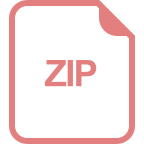